vue3 ts echarts
时间: 2023-11-11 17:03:51 浏览: 36
您的问题是关于 Vue3 和 TypeScript 结合使用 Echarts 的知识吗?
如果是的话,您可以按照以下步骤来实现:
1. 安装 `echarts` 和 `@types/echarts` 两个依赖:
```
npm install echarts @types/echarts -S
```
2. 在 Vue3 组件中引用 Echarts:
```typescript
import { defineComponent } from 'vue';
import * as echarts from 'echarts';
export default defineComponent({
mounted() {
const chartDom = document.getElementById('chart');
const myChart = echarts.init(chartDom);
// 在此处编写 Echarts 相关代码
},
// 省略其他代码
});
```
3. 使用 TypeScript 定义 Echarts 数据:
```typescript
interface Data {
name: string;
value: number;
}
interface Option {
xAxis: {
type: string;
data: string[];
};
yAxis: {
type: string;
};
series: {
name: string;
data: Data[];
type: string;
}[];
}
export default defineComponent({
data() {
return {
option: {} as Option, // 强制类型转换
};
},
mounted() {
const chartDom = document.getElementById('chart');
const myChart = echarts.init(chartDom);
const data = [
{ name: 'A', value: 10 },
{ name: 'B', value: 20 },
{ name: 'C', value: 30 },
{ name: 'D', value: 40 },
{ name: 'E', value: 50 },
];
this.option = {
xAxis: {
type: 'category',
data: data.map(item => item.name),
},
yAxis: {
type: 'value',
},
series: [
{
name: 'Demo',
data: data,
type: 'bar',
},
],
};
myChart.setOption(this.option);
},
// 省略其他代码
});
```
相关推荐
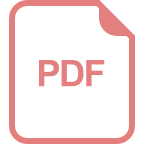














