我有一份数据集,包含很多个按时间排序(每15分钟一次)的hdf5文件,hdf5文件又是包含一个二维数组,我怎么对这个建立模型预测接下来一个小时的数据结果
时间: 2024-11-12 17:35:55 浏览: 27
对于这种时序数据集,你可以使用Python中的NumPy和Pandas库进行预处理,然后利用深度学习框架如TensorFlow或Keras构建模型。以下是步骤概述:
1. **数据加载**:
使用`pandas`的`read_hdf`函数读取hdf5文件,将它们合并成一个大的DataFrame,按照时间顺序排列。如果数据量大,可以考虑分块读取。
```python
import pandas as pd
df = pd.concat([pd.read_hdf(file) for file in hdf5_files], ignore_index=True)
```
2. **数据处理**:
- 将时间戳转换为适合模型的数值表示(例如,通过Unix时间戳或日期差分)。
- 创建目标变量(未来的15分钟或1小时数据),通常这需要将数据划分为训练集和测试集,以及填充或切割数据以保持相同的输入长度。
3. **序列编码**:
对于连续的时序数据,可能需要将其转换为固定长度的序列,可以使用像LSTM、GRU或Transformer这样的循环神经网络架构(RNNs)来处理变长序列。
```python
from sklearn.preprocessing import TimeseriesSplit
tscv = TimeseriesSplit(gap=1) # 每次跨越1个时间段
for train_index, test_index in tscv.split(df):
X_train, y_train = df.iloc[train_index][:-1 * time_window], df.iloc[train_index][-time_window:]
X_test, y_test = df.iloc[test_index][:-1 * time_window], df.iloc[test_index][-time_window:]
```
4. **模型训练**:
构建并训练一个2D卷积神经网络(CNN)加上循环层,或者直接使用专门为时序数据设计的模型,如TCN(Temporal Convolutional Networks)。
```python
from tensorflow.keras.models import Sequential
model = Sequential()
model.add(Conv1D(filters, kernel_size, activation='relu', input_shape=(time_window, features)))
model.add(LSTM(units, return_sequences=True))
model.add(TimeDistributed(Dense(output_features)))
model.compile(loss='mse', optimizer='adam')
```
5. **模型评估与预测**:
训练完成后,在测试集上验证模型性能,然后使用模型对未来一个小时的数据进行预测。
6. **保存和调用**:
训练好的模型可以保存到硬盘,之后加载进行实时预测。
```python
model.fit(X_train, y_train, epochs=num_epochs, batch_size=batch_size)
y_pred = model.predict(X_test)
```
阅读全文
相关推荐
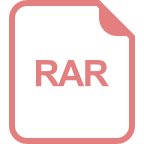
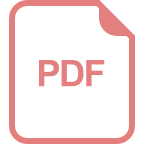
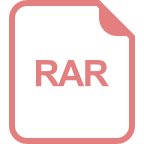
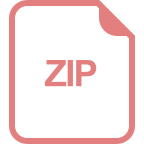
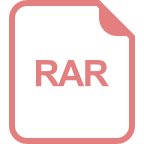
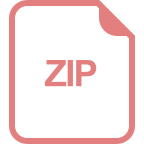
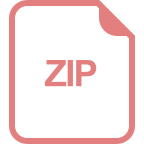
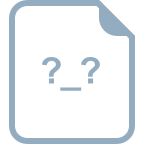
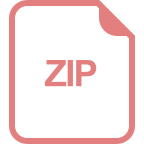
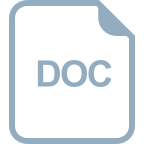
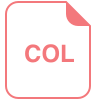
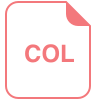






