如何使用jupyter notebook运行Python自动发送邮件
时间: 2024-05-09 20:12:33 浏览: 165
使用Jupyter Notebook运行Python自动发送邮件可以通过以下步骤实现:
1. 安装所需的库:首先,确保你已经安装了`smtplib`和`email`库。如果没有安装,可以使用以下命令进行安装:
```
pip install smtplib
pip install email
```
2. 导入所需的库:在Jupyter Notebook中,首先需要导入`smtplib`和`email`库:
```python
import smtplib
from email.mime.text import MIMEText
from email.header import Header
```
3. 设置发件人和收件人信息:在代码中设置发件人和收件人的信息,包括发件人邮箱、收件人邮箱、主题和正文内容:
```python
sender = 'your_email@example.com'
receiver = 'recipient_email@example.com'
subject = 'Subject of the email'
message = 'Content of the email'
```
4. 创建邮件对象:使用`MIMEText`类创建一个邮件对象,并设置邮件的主题和正文内容:
```python
msg = MIMEText(message, 'plain', 'utf-8')
msg['Subject'] = Header(subject, 'utf-8')
```
5. 登录发件人邮箱:使用`smtplib`库登录发件人的邮箱。你需要提供发件人的邮箱地址和密码:
```python
smtp_server = 'smtp.example.com'
username = 'your_email@example.com'
password = 'your_password'
server = smtplib.SMTP(smtp_server, 25)
server.login(username, password)
```
6. 发送邮件:使用`sendmail`方法发送邮件。你需要提供发件人、收件人和邮件内容:
```python
server.sendmail(sender, receiver, msg.as_string())
```
7. 关闭连接:发送完邮件后,记得关闭与SMTP服务器的连接:
```python
server.quit()
```
这样,你就可以在Jupyter Notebook中使用Python自动发送邮件了。
阅读全文
相关推荐
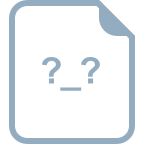
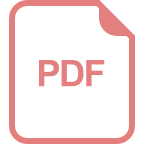
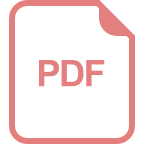
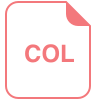
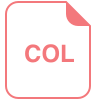
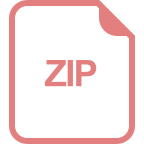
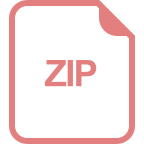
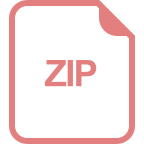
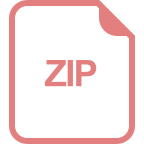
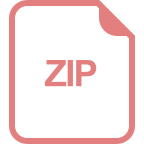
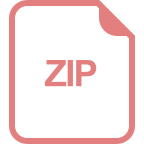
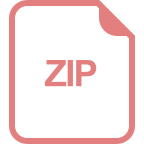
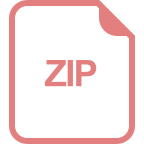
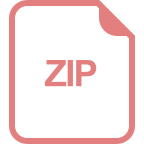
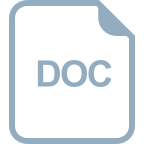
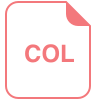
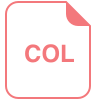
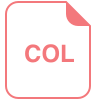
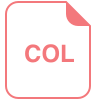