用opengl编写一段程序,实现一个有创意的二维动画
时间: 2024-09-24 21:09:17 浏览: 73
在OpenGL中创建一个创意的二维动画,首先你需要安装并设置好OpenGL环境,然后利用GLUT库或者现代的OpenGL ES进行编程。以下是一个基本步骤示例:
```cpp
#include <GL/glut.h>
#include <cmath>
// 定义一个简单的二维图形结构
class Shape {
public:
virtual void draw() = 0;
};
class Circle : public Shape {
private:
float radius;
public:
Circle(float r) : radius(r) {}
void draw() override {
glBegin(GL圓);
glVertex2f(radius, 0);
glEnd();
}
};
class Square : public Shape {
private:
float side;
public:
Square(float s) : side(s) {}
void draw() override {
glBegin(GL_QUADS);
glVertex2f(-side, -side);
glVertex2f(side, -side);
glVertex2f(side, side);
glVertex2f(-side, side);
glEnd();
}
};
// 主函数
void display() {
glClear(GL_COLOR_BUFFER_BIT);
// 动画示例:圆和正方形交替出现
static int shapeIndex = 0;
if (shapeIndex == 0) {
Circle circle(50);
circle.draw();
} else {
Square square(100);
square.draw();
}
shapeIndex = (shapeIndex + 1) % 2; // 循环切换
glutSwapBuffers();
}
int main(int argc, char** argv) {
glutInit(&argc, argv);
glutCreateWindow("Creative 2D Animation");
glEnable(GL_BLEND); // 设置混合模式
glBlendFunc(GL_SRC_ALPHA, GL_ONE_MINUS_SRC_ALPHA); // 透明度效果
glutDisplayFunc(display);
glutMainLoop();
return 0;
}
```
在这个例子中,我们定义了两个简单的形状(圆形和正方形),它们都继承自`Shape`基类,并实现了`draw()`方法。在`display()`函数中,我们通过改变`shapeIndex`来控制当前显示的是哪一种形状,从而形成一个简单的交替动画。
阅读全文
相关推荐
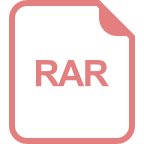
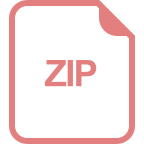
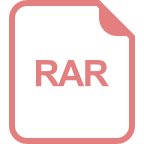
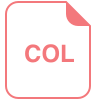
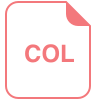
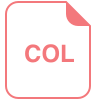
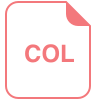
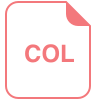
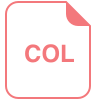
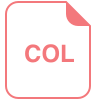
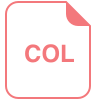
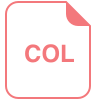
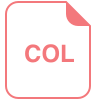
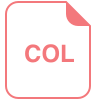