C语言怎么给值设定取值范围
时间: 2024-09-10 15:14:28 浏览: 103
在C语言中,没有直接提供内置的方式来设置变量的取值范围,因为这是由程序员在编写程序时通过数据类型和初始化来控制的。例如:
1. **整型**:对于`int`类型的变量,默认范围通常是-32768到32767(取决于具体的系统,但标准规定了2's complement表示法),可以使用更宽的类型如`long int`或`unsigned int`来扩大范围。
```c
// 示例
signed int value; // 范围通常为 -32768 到 32767 (取决于平台)
unsigned int large_value; // 范围通常从0到2^32-1
```
2. **浮点数**:`float`和`double`有各自的精度限制,`float`约6位有效数字,`double`约15位。
```c
float small_float;
double larger_float;
```
3. **枚举**:虽然不是直接限定了数值范围,但可以自定义一组特定的值。
```c
enum Color {RED, GREEN, BLUE}; // 颜色枚举,取值范围就是预设的三个值
```
为了防止超出范围,可以在程序中进行条件检查和异常处理。然而,C语言本身并不强制要求这样做,错误处理通常由程序员根据具体需求来实现。
相关问题
C语言设定一个 设定一个二元信号量Q,其(1,0)中交替取值
以下是使用C语言实现设定一个二元信号量Q,其(1,0)中交替取值的代码:
```c
#include <stdio.h>
#include <pthread.h>
pthread_mutex_t mutex;
pthread_cond_t cond;
int flag = 0;
void *thread1(void *arg)
{
while(1)
{
pthread_mutex_lock(&mutex);
while(flag != 0)
{
pthread_cond_wait(&cond, &mutex);
}
printf("Thread 1: 1\n");
flag = 1;
pthread_cond_signal(&cond);
pthread_mutex_unlock(&mutex);
}
}
void *thread2(void *arg)
{
while(1)
{
pthread_mutex_lock(&mutex);
while(flag != 1)
{
pthread_cond_wait(&cond, &mutex);
}
printf("Thread 2: 0\n");
flag = 0;
pthread_cond_signal(&cond);
pthread_mutex_unlock(&mutex);
}
}
int main()
{
pthread_t tid1, tid2;
pthread_mutex_init(&mutex, NULL);
pthread_cond_init(&cond, NULL);
pthread_create(&tid1, NULL, thread1, NULL);
pthread_create(&tid2, NULL, thread2, NULL);
pthread_join(tid1, NULL);
pthread_join(tid2, NULL);
pthread_mutex_destroy(&mutex);
pthread_cond_destroy(&cond);
return 0;
}
```
在主函数中创建了两个线程,分别是thread1和thread2。线程1用来打印数字1,线程2用来打印数字0。两个线程通过使用pthread_mutex_t类型的互斥锁mutex和pthread_cond_t类型的条件变量cond来实现同步。变量flag用来表示当前应该打印的数字。
线程1首先获取互斥锁mutex,然后如果flag不等于0,就进入等待状态,直到其它线程发出信号通知它可以继续执行。一旦获取到信号,就打印数字1,将flag设置为1,并发出信号通知其它线程可以继续执行。最后释放互斥锁mutex。
线程2的实现类似,只是它等待的条件是flag等于1,打印数字0,将flag设置为0,然后发出信号通知其它线程可以继续执行。
通过这种方式,就可以实现一个二元信号量Q,其(1,0)中交替取值。
我的设定温度范围是0-350摄氏度,降温设备有1-50个挡位用于降温,挡位越大降温效果越好。使用C语言帮我生成一个完善的PID控制代码用于设备降温。要考虑到各种异常的处理和初始化,并提供一个使用示例。每个函数要详细注释用法和参数取值范围。并注释每一句代码。
在C语言中编写一个完整的PID控制器代码来控制温度是非常复杂的工作,因为它涉及到实时数据采集、数学运算和硬件控制。这里给出一个简化版的基本框架,仅作为一个起点,你可能需要根据实际环境对代码进行修改和完善。以下是部分核心代码片段,包括PID控制结构和异常处理:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
// 定义PID类
typedef struct {
float kp; // 比例增益
float ki; // 积分增益
float kd; // 微分增益
float setpoint; // 设定点
float last_error; // 上一周期误差
float integral; // 积分累加
float derivative; // 微分计算
int current_tap; // 当前档位
} PID;
// 初始化PID控制器
PID* init_PID(float kp, float ki, float kd, float setpoint, int max_tap) {
PID *pid = malloc(sizeof(PID));
pid->kp = kp;
pid->ki = ki;
pid->kd = kd;
pid->setpoint = setpoint;
pid->last_error = 0;
pid->integral = 0;
pid->derivative = 0;
pid->current_tap = 1;
if (max_tap < 1 || max_tap > 50) {
fprintf(stderr, "Invalid maximum tap value.\n");
exit(EXIT_FAILURE);
}
return pid;
}
// 更新PID控制器
void update_PID(PID* pid, float current_temperature, float dt) {
float error = pid->setpoint - current_temperature;
pid->integral += error * dt;
pid->derivative = (error - pid->last_error) / dt;
pid->last_error = error;
// 调整档位
float target_tap = pid->kp * error + pid->ki * pid->integral + pid->kd * pid->derivative;
int new_tap = floor(target_tap + 0.5); // 向上取整,因为档位不能低于1
if (new_tap > pid->current_tap && new_tap <= 50) {
pid->current_tap = new_tap;
} else {
fprintf(stderr, "Temperature control out of range: requested %d, actual %d\n", new_tap, pid->current_tap);
}
}
// 使用示例
int main(int argc, char** argv) {
float kp = 1.0; // 设置比例增益
float ki = 0.05; // 设置积分增益
float kd = 0.1; // 设置微分增益
float setpoint = 250.0; // 设定温度
PID *pid = init_PID(kp, ki, kd, setpoint, 50);
// 假设有一个循环,定期获取温度并更新PID
while (true) {
float temperature = get_current_temperature(); // 从传感器获取实时温度
update_PID(pid, temperature, 0.1); // dt是时间间隔,单位s
apply_tap_to_device(pid->current_tap); // 根据档位控制降温设备
sleep(1); // 模拟执行时间间隔
}
free(pid);
return 0;
}
```
在这个例子中:
- `init_PID` 函数接收比例、积分和微分增益,设定点和最大档位作为输入,返回一个PID实例。
- `update_PID` 函数计算PID控制输出并调整档位,处理可能的异常。
- `main` 函数展示了如何在一个无限循环中使用PID控制器。
注意,这个代码假设了一些不存在的函数如`get_current_temperature()`和`apply_tap_to_device()`,你需要根据实际情况替换成真实的数据获取和设备控制函数。此外,对于实时控制,你需要处理时间差`dt`和线程同步等问题。
阅读全文
相关推荐
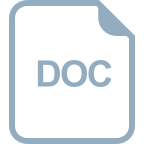
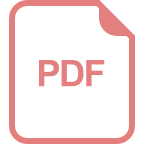
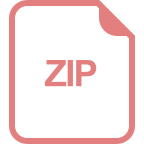
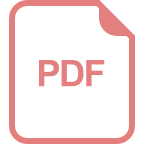
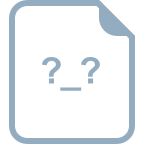
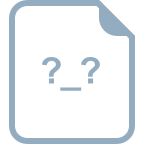
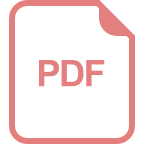
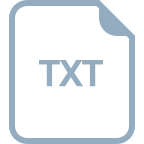
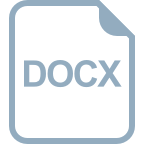
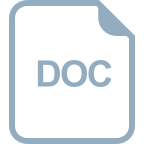
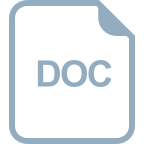




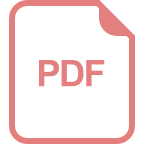