python excel导入数据库
时间: 2023-08-26 15:17:20 浏览: 105
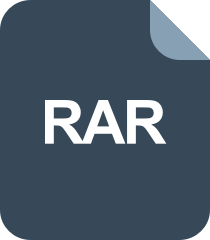
excel导入数据库
要将Excel中的数据导入到数据库中,你可以使用Python中的pandas库和数据库连接库(如pymysql)。首先,你需要使用xlrd库读取Excel文件,并将数据保存到一个列表中。然后,你可以使用pandas库将列表中的数据转换成DataFrame对象。接下来,你可以使用数据库连接库连接到数据库,并将DataFrame中的数据插入到数据库表中。
下面是一个示例代码:
```python
import xlrd
import pymysql
import pandas as pd
# 读取Excel文件
file_path = 'path/to/your/excel/file.xlsx'
workbook = xlrd.open_workbook(file_path)
sheet = workbook.sheet_by_index(0)
data = []
for row in range(sheet.nrows):
data.append(sheet.row_values(row))
# 将数据保存到DataFrame中
df = pd.DataFrame(data)
# 连接数据库
conn = pymysql.connect(host='your_host', user='your_username', password='your_password', db='your_database')
cursor = conn.cursor()
# 插入数据到数据库表中
for index, row in df.iterrows():
sql = "INSERT INTO your_table_name (column1, column2, ...) VALUES (%s, %s, ...)"
values = tuple(row)
cursor.execute(sql, values)
conn.commit()
cursor.close()
conn.close()
print("数据导入成功!")
```
请确保将代码中的`your_host`、`your_username`、`your_password`、`your_database`、`your_table_name`替换为你实际的数据库连接信息和表名。
希望这个示例能帮到你!<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *3* [Python解决 excel导入数据库 & Python读取数据库并保存 excel文件(多种方法&详细讲解)](https://blog.csdn.net/Frost216/article/details/120746026)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
- *2* [Python读取excel文件数据并插入数据库](https://blog.csdn.net/tyh_keephunger/article/details/121970697)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v92^chatsearchT3_1"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
阅读全文
相关推荐

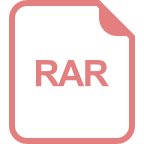
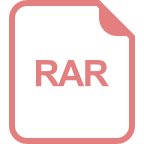
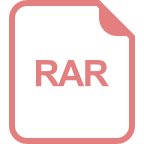









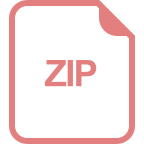
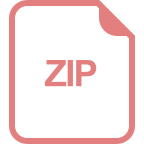