dnn_superres
时间: 2024-12-26 15:14:42 浏览: 5
### DNN 超分辨率实现与技术
#### 深度学习模型用于图像超分辨率
深度卷积神经网络(DCNNs)已被广泛应用于提升低分辨率图像的质量。通过引入残差学习框架,可以有效解决深层网络训练中的梯度消失问题[^1]。
```python
import torch.nn as nn
class ResidualBlock(nn.Module):
def __init__(self, channels):
super(ResidualBlock, self).__init__()
self.conv1 = nn.Conv2d(channels, channels, kernel_size=3, padding=1)
self.bn1 = nn.BatchNorm2d(channels)
self.prelu = nn.PReLU()
self.conv2 = nn.Conv2d(channels, channels, kernel_size=3, padding=1)
self.bn2 = nn.BatchNorm2d(channels)
def forward(self, x):
residual = x
out = self.conv1(x)
out = self.bn1(out)
out = self.prelu(out)
out = self.conv2(out)
out = self.bn2(out)
out += residual
return out
```
#### 子像素卷积层的应用
子像素卷积层能够有效地将特征图放大到高分辨率空间,从而提高重建精度并减少计算量。该方法利用了高效的空间转换操作来替代传统的插值方式。
```python
def pixel_shuffle(input, upscale_factor):
batch_size, channels, in_height, in_width = input.size()
channels //= upscale_factor ** 2
out_height = in_height * upscale_factor
out_width = in_width * upscale_factor
input_view = input.contiguous().view(
batch_size, channels, upscale_factor, upscale_factor,
in_height, in_width
)
shuffle_out = input_view.permute(0, 1, 4, 2, 5, 3).contiguous()
return shuffle_out.view(batch_size, channels, out_height, out_width)
```
#### 训练策略优化
为了获得更好的性能,在训练过程中通常会采用多种损失函数组合的方式来进行监督学习。除了常见的均方误差(MSE),还可以加入感知损失(perceptual loss)以及对抗损失(adversarial loss)。
```python
criterion_mse = nn.MSELoss()
criterion_perceptual = PerceptualLoss() # 自定义或第三方库提供
criterion_adversarial = AdversarialLoss()
total_loss = criterion_mse(output, target) + \
perceptual_weight * criterion_perceptual(output, target) + \
adversarial_weight * criterion_adversarial(discriminator_output)
```
阅读全文
相关推荐
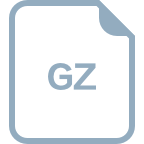











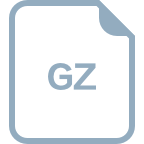





