输入10个学生的学号(整数)和这10个学生的3门课程的成绩(整数),统计并输出3门课程总分最高的学生的学号和总分(整数)。c语言
时间: 2024-12-14 07:17:25 浏览: 4
在C语言中,你可以通过数组和结构体来存储学生信息,然后遍历数组找出总分最高者。以下是一个简单的示例程序:
```c
#include <stdio.h>
// 定义学生结构体
typedef struct {
int student_id; // 学号
int course1_score;
int course2_score;
int course3_score;
} Student;
int main() {
const int num_students = 10;
Student students[num_students];
// 输入学生信息
for (int i = 0; i < num_students; i++) {
printf("请输入第%d个学生的学号和三门课成绩:\n", i + 1);
scanf("%d %d %d %d", &students[i].student_id, &students[i].course1_score,
&students[i].course2_score, &students[i].course3_score);
}
// 初始化最高分变量
int highest_score = 0;
int max_student_id;
// 遍历学生数组,比较总分
for (int i = 0; i < num_students; i++) {
int total_score = students[i].course1_score + students[i].course2_score + students[i].course3_score;
if (total_score > highest_score) {
highest_score = total_score;
max_student_id = students[i].student_id;
}
}
// 输出结果
printf("总分最高的学生学号是%d,总分为:%d。\n", max_student_id, highest_score);
return 0;
}
```
阅读全文
相关推荐
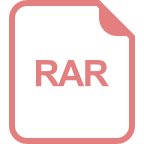
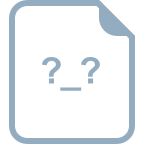
















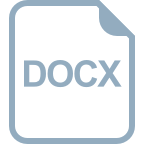