银行家算法找安全序列
时间: 2024-01-03 19:04:50 浏览: 77
银行家算法是一种用于避免死锁的算法,它通过判断系统是否处于安全状态来决定是否分配资源。以下是银行家算法找到安全序列的步骤:
1. 初始化:将所有进程的完成状态设置为False,将所有资源的可用数量和进程的需求资源数量记录下来。
2. 找到一个未完成的进程P,满足以下条件:
- 进程P的需求资源数量小等于可用资源数量。
- 进程P所需的资源数量减去已分配给它的资源数量后,不会导致系统进入不安全状态。
3. 将进程P标记为已完成,并释放进程P所占用的资源。
4. 更新可用资源数量:将进程P释放的资源数量加回可用资源数量。
5. 重复步骤2和步骤3,直到找不到满足条件的进程。
如果找到了一个安全序列,即所有进程都能够完成并释放资源,那么系统就处于安全状态。否则,系统处于不安全状态。
以下是一个银行家算法找到安全序列的示例代码:
```cpp
#include <iostream>
#include <vector>
using namespace std;
bool isSafe(vector<int> available, vector<vector<int>> max, vector<vector<int>> allocation) {
int numProcesses = max.size();
int numResources = available.size();
vector<int> work = available;
vector<bool> finish(numProcesses, false);
vector<int> safeSequence;
int count = 0;
while (count < numProcesses) {
bool found = false;
for (int i = 0; i < numProcesses; i++) {
if (!finish[i]) {
bool canAllocate = true;
for (int j = 0; j < numResources; j++) {
if (max[i][j] - allocation[i][j] > work[j]) {
canAllocate = false;
break;
}
}
if (canAllocate) {
for (int j = 0; j < numResources; j++) {
work[j] += allocation[i][j];
}
safeSequence.push_back(i);
finish[i] = true;
found = true;
count++;
}
}
}
if (!found) {
break;
}
}
if (count == numProcesses) {
cout << "System is in safe state." << endl;
cout << "Safe sequence: ";
for (int i = 0; i < numProcesses; i++) {
cout << safeSequence[i] << " ";
}
cout << endl;
return true;
} else {
cout << "System is in unsafe state." << endl;
return false;
}
}
int main() {
vector<int> available = {3, 3, 2};
vector<vector<int>> max = {
{7, 5, 3},
{3, 2, 2},
{9, 0, 2},
{2, 2, 2},
{4, 3, 3}
};
vector<vector<int>> allocation = {
{0, 1, 0},
{2, 0, 0},
{3, 0, 2},
{2, 1, 1},
{0, 0, 2}
};
isSafe(available, max, allocation);
return 0;
}
```
相关推荐
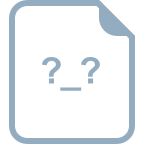
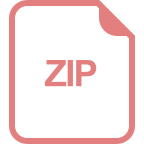
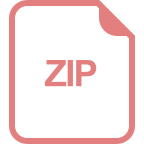
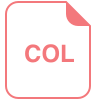
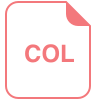
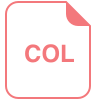
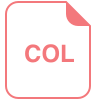
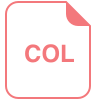









