python asyncio 协程 socket 客户端
时间: 2024-08-04 14:00:36 浏览: 164
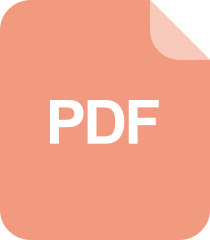
Python之进程+线程+协程(异步、selectors模块、阻塞、非阻塞IO)
Python asyncio 是一个用于编写高性能并发代码的模块,特别适合处理I/O密集型任务,如网络编程。使用 asyncio 和 sockets 可以创建高效的异步客户端,它可以同时处理多个连接请求,而不会阻塞主线程。
以下是一个简单的 asyncio Socket 客户端示例,它使用 `asyncio` 的 `create_task`、`connect()` 函数以及 `StreamReader` 和 `StreamWriter` 来与服务器进行异步通信:
```python
import asyncio
import aiohttp
import socket
async def async_client(message, host='localhost', port=8000):
reader, writer = await asyncio.open_connection(host, port)
print(f"Connected to server: {host}:{port}")
# Write message to the server
writer.write(message.encode())
await writer.drain() # Ensure the message is sent before reading
# Read response from the server
response = await reader.readuntil(b'\n')
print(f"Received from server: {response.decode()}")
# Close the connection
writer.close()
await writer.wait_closed()
async def main():
message = "Hello, Server!"
await async_client(message)
if __name__ == "__main__":
asyncio.run(main())
```
在这个例子中,`async_client` 函数创建了一个异步连接,发送消息,接收响应,并关闭连接。`main` 函数启动了客户端的异步操作。
相关问题:
1. 在异步客户端中,`await open_connection()` 返回的是什么?
2. `reader.readuntil()` 函数的作用是什么?
3. 如何确保在读取服务器响应之前,消息已经完全发送到服务器?
4. 异步客户端如何处理可能出现的连接错误?
阅读全文
相关推荐
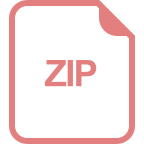
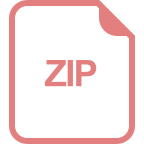
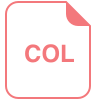
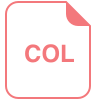
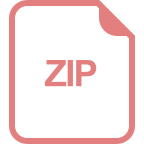
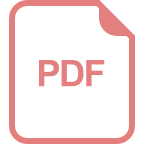
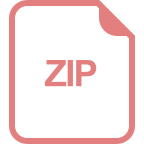
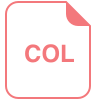
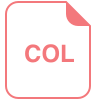
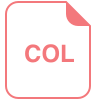
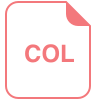
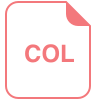
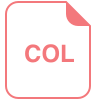




