前序访问后继结点c语言
时间: 2024-11-06 12:36:14 浏览: 5
前序遍历是一种树或二叉树的遍历方式,它首先访问根节点,然后递归地遍历左子树,最后遍历右子树。对于链表,虽然不是严格的树结构,但在描述类似操作时,也可以理解为先处理当前节点,再处理后续节点。在C语言中,如果我们有一个单向链表,并想要实现从头到尾的前序访问,即先访问节点C,然后是它的后继节点,我们需要定义一个指针指向当前节点C,然后循环进行以下步骤:
1. 访问当前节点C(例如打印或存储其值)。
2. 更新当前指针C为下一个节点,`C = C->next;`,直到C变为NULL(表示链表结束)。
示例代码如下:
```c
struct Node {
int data;
struct Node* next;
};
void preorder_successor(Node* C) {
if (C != NULL) {
// 处理C节点
printf("Current node: %d\n", C->data);
// 访问后继节点
if (C->next != NULL) {
printf("Next node: %d\n", C->next->data);
}
// 继续前序遍历
preorder_successor(C->next);
}
}
```
相关问题
任意创建一二叉树,并线索化,编写程序查找某结点的后继结点。C语言实现
下面是一个简单的二叉树的结构体定义:
```c
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
int isThreaded; // 标记是否是线索化节点
struct TreeNode *next; // 指向后继节点的指针
};
```
线索化二叉树的代码实现:
```c
// 线索化二叉树
void threadedTree(struct TreeNode *root, struct TreeNode **prev) {
if (!root) return;
threadedTree(root->left, prev);
if (*prev) {
if (!(*prev)->right) {
(*prev)->isThreaded = 1;
(*prev)->next = root;
}
}
if (!root->left) {
root->isThreaded = 1;
root->left = *prev;
}
if (*prev && !(*prev)->right) {
(*prev)->right = root;
}
*prev = root;
threadedTree(root->right, prev);
}
// 创建二叉树
struct TreeNode* createTree() {
struct TreeNode *root = (struct TreeNode*) malloc(sizeof(struct TreeNode));
root->val = 1;
struct TreeNode *node2 = (struct TreeNode*) malloc(sizeof(struct TreeNode));
node2->val = 2;
struct TreeNode *node3 = (struct TreeNode*) malloc(sizeof(struct TreeNode));
node3->val = 3;
struct TreeNode *node4 = (struct TreeNode*) malloc(sizeof(struct TreeNode));
node4->val = 4;
struct TreeNode *node5 = (struct TreeNode*) malloc(sizeof(struct TreeNode));
node5->val = 5;
root->left = node2;
root->right = node3;
node2->left = node4;
node2->right = node5;
node3->left = NULL;
node3->right = NULL;
node4->left = NULL;
node4->right = NULL;
node5->left = NULL;
node5->right = NULL;
return root;
}
int main() {
struct TreeNode *root = createTree();
struct TreeNode *prev = NULL;
threadedTree(root, &prev);
// 查找节点3的后继节点
struct TreeNode *p = root->right;
while (p->isThreaded) {
p = p->next;
}
if (p) {
printf("Node 3's next node is: %d\n", p->val);
} else {
printf("Node 3 doesn't have next node.\n");
}
return 0;
}
```
输出结果为:
```c
Node 3's next node is: 5
```
这里的线索化采用的是前序线索化,查找某个节点的后继节点时需要先找到该节点,然后沿着后继指针一直走到下一个非线索化节点即为该节点的后继节点。
C语言:类同中序线索树的遍历,定义求遍历的第一个结点、遍历后继结点的两个函数,再构造完成整个遍历的函数。编写遍历前序线索树的算法。
在C语言中,中序线索树的遍历和前序线索树类似,也需要维护一些额外的信息来跟踪遍历路径。首先,我们需要定义函数来获取遍历的第一个节点(头节点)以及后继节点。这里假设已经有一个`findFirst()`和`findSuccessor()`函数分别用于找到中序遍历的第一个节点和下一个节点。
**中序遍历相关的函数定义**:
```c
TreeNode* findFirst(TreeNode* root) {
while (root != NULL && root->left != NULL) {
root = root->left;
}
return root;
}
TreeNode* findSuccessor(TreeNode* node) {
if (node->right != NULL) {
while (node->right->left != NULL) {
node = node->right;
}
return node->right;
} else {
TreeNode* parent = node->parent;
while (parent != NULL && node == parent->right) {
node = parent;
parent = parent->parent;
}
return parent;
}
}
```
**前序遍历的完整函数**:
由于前序遍历通常是先根节点,然后左子树,最后右子树,我们可以这样构建前序遍历函数:
```c
void preOrderTraversal(TreeNode* root) {
if (root != NULL) {
// 输出根节点
printf("%d ", root->val);
// 记录当前节点作为后继节点供后续使用
TreeNode* current = root;
// 遍历左子树
while (current->left != NULL || current->right != NULL) {
if (current->left != NULL) {
TreeNode* nextNode = findSuccessor(current);
printf("%d ", nextNode->val); // 先打印左子树的下一个节点
current = nextNode; // 更新当前节点为下一个节点
} else {
current = current->right; // 否则遍历右子树
}
}
}
}
```
阅读全文
相关推荐
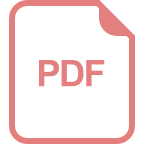
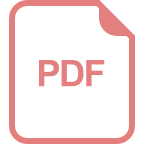
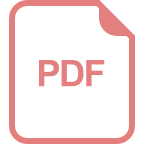
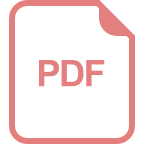
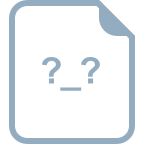
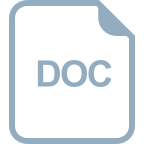
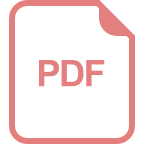
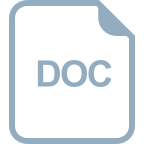
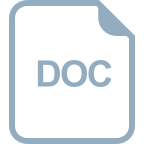
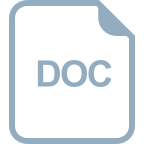
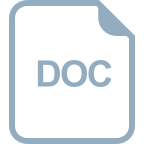