编写一个程序,编写一个python源程序文件,将文件中所有除保留字外的小写字母换成大写字母,生成后的文件要能够被python解释器正确执行。
时间: 2024-10-14 08:06:50 浏览: 23
为了编写这样一个Python程序,你可以使用内置的`open()`, `read()`, `replace()`, 和 `write()` 函数。以下是一个简单的步骤说明:
1. 打开原始文件,假设文件名为`input.txt`。
2. 读取文件内容到一个字符串变量中。
3. 使用正则表达式或`str.islower()`检查每个字符,如果它是小写字母且不是保留字(Python的保留字不需要转换),就将其替换为大写字母。
4. 将处理过的字符串写入新的文件,例如`output.txt`。
5. 关闭文件。
下面是一个示例代码:
```python
import re
# Python的保留字列表
RESERVED_WORDS = {
'and', 'as', 'assert', 'async', 'await', 'break', 'class', 'continue',
'def', 'del', 'elif', 'else', 'except', 'False', 'finally', 'for',
'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not',
'or', 'pass', 'raise', 'return', 'True', 'try', 'while', 'with', 'yield'
}
def process_file(input_file, output_file):
with open(input_file, 'r') as f_in, open(output_file, 'w') as f_out:
content = f_in.read()
# 使用正则表达式匹配非保留字的小写字母并替换为大写
content = re.sub(r'(?<!\b)\w[^\W\d_]*[a-z]', lambda m: m.group().upper(), content)
for word in RESERVED_WORDS:
if content.find(word) != -1 and content[word].islower():
content = content.replace(word, word.upper())
f_out.write(content)
# 调用函数处理文件
process_file('input.txt', 'output.txt')
```
运行此程序后,`input.txt` 文件中的小写字母(非保留字)会被转换为大写字母,并保存到 `output.txt` 中。
阅读全文
相关推荐
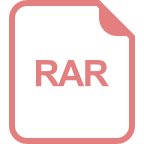
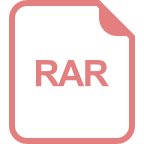
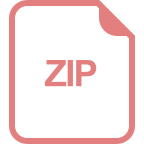








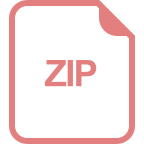
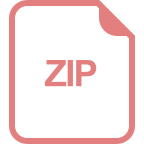
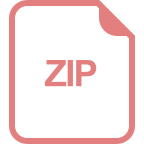
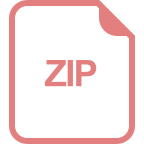
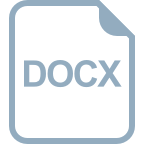
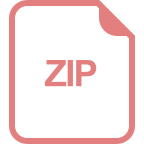
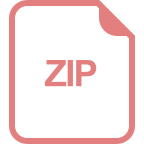
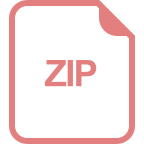