unordered_map能不能用pii
时间: 2024-03-29 10:33:18 浏览: 24
unordered_map是C++标准库中的一个容器,用于存储键值对。其中的键和值可以是任意类型,包括pii(pair<int, int>)类型。
unordered_map可以使用pii作为键或值,只要pii类型满足unordered_map的要求即可。unordered_map的键类型需要满足可哈希化的要求,即需要提供一个哈希函数和相等比较函数。对于pii类型,默认情况下是不满足这个要求的,因为pii类型没有默认的哈希函数和相等比较函数。
但是,我们可以自定义pii类型的哈希函数和相等比较函数,然后将其作为unordered_map的键或值。例如,可以使用std::hash和std::equal_to来定义pii类型的哈希函数和相等比较函数。
下面是一个示例代码:
```cpp
#include <iostream>
#include <unordered_map>
#include <utility>
struct PiiHash {
std::size_t operator()(const std::pair<int, int>& pii) const {
return std::hash<int>()(pii.first) ^ std::hash<int>()(pii.second);
}
};
struct PiiEqual {
bool operator()(const std::pair<int, int>& lhs, const std::pair<int, int>& rhs) const {
return lhs.first == rhs.first && lhs.second == rhs.second;
}
};
int main() {
std::unordered_map<std::pair<int, int>, int, PiiHash, PiiEqual> map;
map[{1, 2}] = 3;
map[{4, 5}] = 6;
std::cout << map[{1, 2}] << std::endl; // 输出3
std::cout << map[{4, 5}] << std::endl; // 输出6
return 0;
}
```
在上面的示例中,我们定义了一个PiiHash结构体和一个PiiEqual结构体,分别作为pii类型的哈希函数和相等比较函数。然后,我们使用这两个结构体作为unordered_map的模板参数,来创建一个以pii为键的unordered_map。
相关推荐
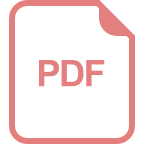
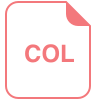
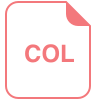
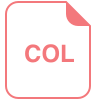
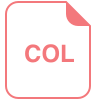
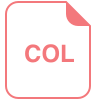









