hdfs上传文件代码
时间: 2024-06-24 08:01:34 浏览: 157
在Hadoop分布式文件系统(HDFS)中,上传文件通常使用Hadoop的命令行工具Hadoop File System (HDFS) Client,或者通过编程方式使用Hadoop的API。这里我将演示一个基本的通过Hadoop命令行上传文件的步骤[^4]:
```shell
# 假设你已经登录到Hadoop集群并切换到HDFS用户
hadoop fs -put <本地文件路径> <HDFS文件路径>
```
例如,如果你要将本地的`/home/user/myfile.txt`上传到HDFS的`/user/hdfs/destination`目录,命令会是:
```shell
hadoop fs -put /home/user/myfile.txt /user/hdfs/destination/myfile.txt
```
这将会把`myfile.txt`复制到HDFS指定的位置。
如果你想通过编程方式,如Java,可以使用`FileSystem`接口[^5]。例如:
```java
Configuration conf = new Configuration();
FileSystem fs = FileSystem.get(conf);
Path localFile = new Path("/path/to/local/file");
Path hdfsFile = new Path("/path/in/hdfs/file");
fs.copyFromLocalFile(localFile, hdfsFile);
```
相关问题
hdfs上传文件的代码
在Hadoop分布式文件系统(HDFS)上上传文件通常涉及到使用Hadoop的API或者命令行工具`hadoop fs`。这里是一个简单的Java示例,展示了如何使用Apache Hadoop的FileSystem API来上传文件:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
public class HdfsFileUploader {
public static void main(String[] args) throws Exception {
// 设置HDFS配置
Configuration conf = new Configuration();
String hdfsUrl = "your_hdfs_namenode_url"; // 例如:hdfs://localhost:9000
FileSystem fs = FileSystem.get(conf);
// 指定要上传的本地文件路径
Path localFilePath = new Path("path_to_your_local_file");
// 指定HDFS的目标路径
Path hdfsTargetPath = new Path(hdfsUrl + "/path/to/upload/in/hdfs");
// 使用FSDataOutputStream将文件写入HDFS
fs.copyFromLocalFile(localFilePath, hdfsTargetPath);
System.out.println("File uploaded successfully to " + hdfsTargetPath);
fs.close(); // 关闭连接
}
}
```
在这个例子中,你需要替换`your_hdfs_namenode_url`为你实际Hadoop集群的NameNode地址,并指定本地文件和目标HDFS路径。
如果你使用的是命令行工具,你可以这样做:
```bash
hadoop dfs -put /path/to/local/file /path/to/upload/in/hdfs
```
这会将`local/file`复制到HDFS的`/path/to/upload/in/hdfs`目录下。
ssm查看hdfs文件,上传文件,下载文件,删除文件前后端代码
### 回答1:
以下是SSM框架中查看、上传、下载、删除HDFS文件的前后端代码。
### 查看HDFS文件
前端代码:
```html
<!-- index.jsp -->
<form id="form" enctype="multipart/form-data" method="post" action="showFile">
<label for="path">文件路径:</label>
<input type="text" name="path" id="path">
<input type="submit" value="查看">
</form>
```
后端代码:
```java
// FileController.java
@Controller
public class FileController {
private final FileSystem fileSystem = HdfsUtil.getFileSystem();
@RequestMapping("showFile")
@ResponseBody
public String showFile(@RequestParam("path") String path) {
try {
FSDataInputStream inputStream = fileSystem.open(new Path(path));
BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream));
StringBuilder builder = new StringBuilder();
String line = null;
while ((line = reader.readLine()) != null) {
builder.append(line).append("\n");
}
reader.close();
inputStream.close();
return builder.toString();
} catch (IOException e) {
e.printStackTrace();
return "读取文件失败!";
}
}
}
```
### 上传HDFS文件
前端代码:
```html
<!-- index.jsp -->
<form id="form" enctype="multipart/form-data" method="post" action="uploadFile">
<label for="file">上传文件:</label>
<input type="file" name="file" id="file">
<input type="submit" value="上传">
</form>
```
后端代码:
```java
// FileController.java
@Controller
public class FileController {
private final FileSystem fileSystem = HdfsUtil.getFileSystem();
@RequestMapping("uploadFile")
@ResponseBody
public String uploadFile(@RequestParam("file") MultipartFile file) {
try {
String fileName = file.getOriginalFilename();
Path path = new Path("/" + fileName);
FSDataOutputStream outputStream = fileSystem.create(path);
outputStream.write(file.getBytes());
outputStream.close();
return "文件上传成功!";
} catch (IOException e) {
e.printStackTrace();
return "文件上传失败!";
}
}
}
```
### 下载HDFS文件
前端代码:
```html
<!-- index.jsp -->
<form id="form" enctype="multipart/form-data" method="post" action="downloadFile">
<label for="path">文件路径:</label>
<input type="text" name="path" id="path">
<input type="submit" value="下载">
</form>
```
后端代码:
```java
// FileController.java
@Controller
public class FileController {
private final FileSystem fileSystem = HdfsUtil.getFileSystem();
@RequestMapping("downloadFile")
public void downloadFile(@RequestParam("path") String path, HttpServletResponse response) {
try {
FSDataInputStream inputStream = fileSystem.open(new Path(path));
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment; filename=" + new Path(path).getName());
IOUtils.copyBytes(inputStream, response.getOutputStream(), 4096, false);
inputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
### 删除HDFS文件
前端代码:
```html
<!-- index.jsp -->
<form id="form" enctype="multipart/form-data" method="post" action="deleteFile">
<label for="path">文件路径:</label>
<input type="text" name="path" id="path">
<input type="submit" value="删除">
</form>
```
后端代码:
```java
// FileController.java
@Controller
public class FileController {
private final FileSystem fileSystem = HdfsUtil.getFileSystem();
@RequestMapping("deleteFile")
@ResponseBody
public String deleteFile(@RequestParam("path") String path) {
try {
boolean result = fileSystem.delete(new Path(path), true);
return result ? "文件删除成功!" : "文件删除失败!";
} catch (IOException e) {
e.printStackTrace();
return "文件删除失败!";
}
}
}
```
其中,`HdfsUtil`是一个工具类,用于获取HDFS的FileSystem实例。具体实现可参考以下代码:
```java
// HdfsUtil.java
public class HdfsUtil {
private static final String HDFS_URI = "hdfs://localhost:9000";
private static final Configuration CONFIGURATION = new Configuration();
private static FileSystem fileSystem;
static {
try {
fileSystem = FileSystem.get(URI.create(HDFS_URI), CONFIGURATION);
} catch (IOException e) {
e.printStackTrace();
}
}
public static FileSystem getFileSystem() {
return fileSystem;
}
}
```
### 回答2:
为了实现SSM查看HDFS文件、上传文件、下载文件、删除文件的功能,我们需要在前后端代码中分别实现相应的操作。
首先,后端代码:
1. 查看HDFS文件:
```java
@Controller
@RequestMapping("/hdfs")
public class HdfsController {
@Autowired
private HdfsService hdfsService;
@GetMapping("/view")
@ResponseBody
public List<String> viewHdfsFiles() {
return hdfsService.getHdfsFiles();
}
}
```
2. 上传文件:
```java
@Controller
@RequestMapping("/hdfs")
public class HdfsController {
@Autowired
private HdfsService hdfsService;
@PostMapping("/upload")
@ResponseBody
public String uploadFile(@RequestParam("file") MultipartFile file) {
return hdfsService.uploadFile(file);
}
}
```
3. 下载文件:
```java
@Controller
@RequestMapping("/hdfs")
public class HdfsController {
@Autowired
private HdfsService hdfsService;
@GetMapping("/download")
public ResponseEntity<Resource> downloadFile(@RequestParam("path") String path) {
return hdfsService.downloadFile(path);
}
}
```
4. 删除文件:
```java
@Controller
@RequestMapping("/hdfs")
public class HdfsController {
@Autowired
private HdfsService hdfsService;
@GetMapping("/delete")
@ResponseBody
public String deleteFile(@RequestParam("path") String path) {
return hdfsService.deleteFile(path);
}
}
```
然后,前端代码:
1. 查看HDFS文件:
```html
<script>
$(document).ready(function() {
$.ajax({
url: "/hdfs/view",
type: "GET",
success: function(data) {
// 处理返回的文件列表data
}
});
});
</script>
```
2. 上传文件:
```html
<form id="uploadForm" method="POST" enctype="multipart/form-data">
<input type="file" name="file" accept=".txt,.csv">
<button type="submit">上传</button>
</form>
<script>
$("#uploadForm").submit(function(e) {
e.preventDefault();
var formData = new FormData(this);
$.ajax({
url: "/hdfs/upload",
type: "POST",
data: formData,
contentType: false,
processData: false,
success: function(data) {
// 处理上传结果data
}
});
});
</script>
```
3. 下载文件:
```html
<input type="text" id="downloadPath">
<button onclick="downloadFile()">下载</button>
<script>
function downloadFile() {
var path = $("#downloadPath").val();
window.open("/hdfs/download?path=" + path);
}
</script>
```
4. 删除文件:
```html
<input type="text" id="deletePath">
<button onclick="deleteFile()">删除</button>
<script>
function deleteFile() {
var path = $("#deletePath").val();
$.ajax({
url: "/hdfs/delete?path=" + path,
type: "GET",
success: function(data) {
// 处理删除结果data
}
});
}
</script>
```
以上就是SSM查看HDFS文件、上传文件、下载文件、删除文件的前后端代码实现。请根据实际情况进行适当的修改和调整。
### 回答3:
以下是一个简单的SSM项目的前后端代码,实现了查看HDFS文件、上传文件、下载文件、删除文件的功能。
后端代码:
1. HDFSUtil.java
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.springframework.stereotype.Component;
import java.io.InputStream;
import java.io.OutputStream;
@Component
public class HDFSUtil {
private Configuration configuration;
private FileSystem fileSystem;
public HDFSUtil() throws Exception {
configuration = new Configuration();
configuration.set("fs.defaultFS", "hdfs://localhost:9000"); // 设置HDFS地址
fileSystem = FileSystem.get(configuration);
}
public void close() throws Exception {
if (fileSystem != null) {
fileSystem.close();
}
}
public void uploadFile(InputStream inputStream, String destPath) throws Exception {
OutputStream outputStream = fileSystem.create(new Path(destPath));
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) > 0) {
outputStream.write(buffer, 0, length);
}
outputStream.close();
inputStream.close();
}
public void downloadFile(String srcPath, OutputStream outputStream) throws Exception {
InputStream inputStream = fileSystem.open(new Path(srcPath));
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) > 0) {
outputStream.write(buffer, 0, length);
}
outputStream.close();
inputStream.close();
}
public void deleteFile(String path) throws Exception {
fileSystem.delete(new Path(path), true);
}
}
```
2. FileController.java
```java
import org.apache.commons.io.IOUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
@Controller
@RequestMapping("/file")
public class FileController {
@Autowired
private HDFSUtil hdfsUtil;
@RequestMapping(value = "/upload", method = RequestMethod.POST)
@ResponseBody
public String uploadFile(@RequestParam("file") MultipartFile file) {
try {
InputStream inputStream = file.getInputStream();
hdfsUtil.uploadFile(inputStream, "/hdfs/path/" + file.getOriginalFilename());
inputStream.close();
return "上传成功";
} catch (Exception e) {
e.printStackTrace();
return "上传失败";
}
}
@RequestMapping(value = "/download", method = RequestMethod.GET)
public void downloadFile(@RequestParam("path") String path, HttpServletResponse response) {
try {
OutputStream outputStream = response.getOutputStream();
response.setContentType("application/octet-stream");
response.setHeader("Content-Disposition", "attachment; filename=\"" + path + "\"");
hdfsUtil.downloadFile("/hdfs/path/" + path, outputStream);
outputStream.flush();
outputStream.close();
} catch (Exception e) {
e.printStackTrace();
}
}
@RequestMapping(value = "/delete", method = RequestMethod.DELETE)
@ResponseBody
public String deleteFile(@RequestParam("path") String path) {
try {
hdfsUtil.deleteFile("/hdfs/path/" + path);
return "删除成功";
} catch (Exception e) {
e.printStackTrace();
return "删除失败";
}
}
@RequestMapping(value = "/list", method = RequestMethod.GET)
@ResponseBody
public String listFiles() {
try {
// 使用HDFS API获取文件列表
// ...
return "文件列表";
} catch (Exception e) {
e.printStackTrace();
return "获取文件列表失败";
}
}
}
```
前端代码:
1. upload.html
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>HDFS文件上传</title>
</head>
<body>
<form method="post" enctype="multipart/form-data" action="/file/upload">
<input type="file" name="file"/>
<button type="submit">上传</button>
</form>
</body>
</html>
```
2. download.html
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>HDFS文件下载</title>
</head>
<body>
<a href="/file/download?path=filename">下载</a>
</body>
</html>
```
3. delete.html
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>HDFS文件删除</title>
</head>
<body>
<form method="post" action="/file/delete">
<input type="text" name="path"/>
<button type="submit">删除</button>
</form>
</body>
</html>
```
注意:上述代码中的文件路径 "/hdfs/path/" 需要根据实际的HDFS路径进行修改。另外,还需要添加相应的依赖,如Spring MVC、Spring、MyBatis、Hadoop HDFS等。
阅读全文
相关推荐







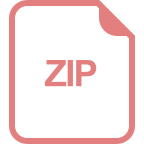
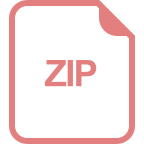
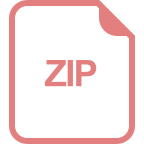