ssm展示HDFS文件列表,上传文件,删除文件,下载文件前后代码
时间: 2023-06-13 10:05:05 浏览: 115
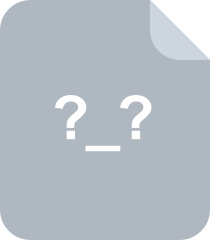
HDFS文件的查看
1. 展示HDFS文件列表
Controller层代码:
```java
@Controller
@RequestMapping("/hdfs")
public class HDFSController {
@Autowired
private HdfsService hdfsService;
// 展示HDFS文件列表
@RequestMapping("/list")
public String list(Model model) throws Exception {
List<String> fileList = hdfsService.listFiles("/");
model.addAttribute("fileList", fileList);
return "hdfs/list";
}
// ...
}
```
Service层代码:
```java
@Service
public class HdfsServiceImpl implements HdfsService {
@Autowired
private FileSystem fileSystem;
// 列出HDFS文件列表
@Override
public List<String> listFiles(String path) throws Exception {
List<String> fileList = new ArrayList<>();
RemoteIterator<LocatedFileStatus> files = fileSystem.listFiles(new Path(path), true);
while (files.hasNext()) {
LocatedFileStatus file = files.next();
fileList.add(file.getPath().toString());
}
return fileList;
}
// ...
}
```
2. 上传文件
Controller层代码:
```java
@Controller
@RequestMapping("/hdfs")
public class HDFSController {
@Autowired
private HdfsService hdfsService;
// 上传文件
@PostMapping("/upload")
public String upload(@RequestParam("file") MultipartFile file, RedirectAttributes redirectAttributes) throws Exception {
if (file.isEmpty()) {
redirectAttributes.addFlashAttribute("message", "Please select a file to upload");
return "redirect:/hdfs/list";
}
String fileName = file.getOriginalFilename();
byte[] bytes = file.getBytes();
hdfsService.uploadFile(bytes, "/" + fileName);
redirectAttributes.addFlashAttribute("message", "You successfully uploaded '" + fileName + "'");
return "redirect:/hdfs/list";
}
// ...
}
```
Service层代码:
```java
@Service
public class HdfsServiceImpl implements HdfsService {
@Autowired
private FileSystem fileSystem;
// 上传文件
@Override
public void uploadFile(byte[] bytes, String path) throws Exception {
FSDataOutputStream outputStream = fileSystem.create(new Path(path));
outputStream.write(bytes);
outputStream.flush();
outputStream.close();
}
// ...
}
```
3. 删除文件
Controller层代码:
```java
@Controller
@RequestMapping("/hdfs")
public class HDFSController {
@Autowired
private HdfsService hdfsService;
// 删除文件
@GetMapping("/delete")
public String delete(@RequestParam("path") String path, RedirectAttributes redirectAttributes) throws Exception {
hdfsService.deleteFile(path);
redirectAttributes.addFlashAttribute("message", "You successfully deleted '" + path + "'");
return "redirect:/hdfs/list";
}
// ...
}
```
Service层代码:
```java
@Service
public class HdfsServiceImpl implements HdfsService {
@Autowired
private FileSystem fileSystem;
// 删除文件
@Override
public void deleteFile(String path) throws Exception {
fileSystem.delete(new Path(path), true);
}
// ...
}
```
4. 下载文件
Controller层代码:
```java
@Controller
@RequestMapping("/hdfs")
public class HDFSController {
@Autowired
private HdfsService hdfsService;
// 下载文件
@GetMapping("/download")
public ResponseEntity<byte[]> download(@RequestParam("path") String path) throws Exception {
byte[] bytes = hdfsService.downloadFile(path);
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", new File(path).getName());
headers.setContentLength(bytes.length);
return new ResponseEntity<>(bytes, headers, HttpStatus.OK);
}
// ...
}
```
Service层代码:
```java
@Service
public class HdfsServiceImpl implements HdfsService {
@Autowired
private FileSystem fileSystem;
// 下载文件
@Override
public byte[] downloadFile(String path) throws Exception {
FSDataInputStream inputStream = fileSystem.open(new Path(path));
byte[] bytes = IOUtils.toByteArray(inputStream);
inputStream.close();
return bytes;
}
// ...
}
```
阅读全文
相关推荐




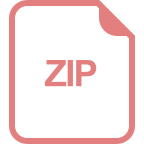
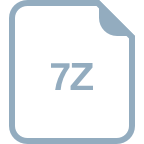
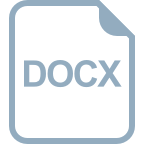
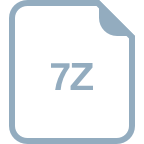