ssm展示HDFS文件列表,上传文件,删除文件,下载文件前后端代码
时间: 2023-06-13 12:04:56 浏览: 61
由于代码较长,这里只提供前端代码的主要部分,后端代码可根据具体项目需求自行编写。
前端代码:
1. 展示HDFS文件列表
```
<template>
<div>
<h3>HDFS文件列表</h3>
<table class="table table-striped">
<thead>
<tr>
<th>文件名</th>
<th>大小</th>
<th>权限</th>
<th>所属用户</th>
</tr>
</thead>
<tbody>
<tr v-for="file in fileList">
<td>{{ file.pathSuffix }}</td>
<td>{{ file.length }}</td>
<td>{{ file.permission }}</td>
<td>{{ file.owner }}</td>
</tr>
</tbody>
</table>
</div>
</template>
<script>
export default {
data() {
return {
fileList: [],
};
},
methods: {
getFileList() {
this.$http.get('/hdfs/list').then((response) => {
this.fileList = response.data;
});
},
},
mounted() {
this.getFileList();
},
};
</script>
```
2. 上传文件
```
<template>
<div>
<h3>上传文件</h3>
<input type="file" @change="uploadFile" />
</div>
</template>
<script>
export default {
methods: {
uploadFile(event) {
const formData = new FormData();
formData.append('file', event.target.files[0]);
this.$http.post('/hdfs/upload', formData).then((response) => {
console.log(response.data);
});
},
},
};
</script>
```
3. 删除文件
```
<template>
<div>
<h3>删除文件</h3>
<input type="text" v-model="path" placeholder="请输入要删除的文件路径" />
<button @click="deleteFile">删除</button>
</div>
</template>
<script>
export default {
data() {
return {
path: '',
};
},
methods: {
deleteFile() {
this.$http.delete(`/hdfs/delete?path=${this.path}`).then((response) => {
console.log(response.data);
});
},
},
};
</script>
```
4. 下载文件
```
<template>
<div>
<h3>下载文件</h3>
<input type="text" v-model="path" placeholder="请输入要下载的文件路径" />
<a :href="`/hdfs/download?path=${path}`" download>下载</a>
</div>
</template>
<script>
export default {
data() {
return {
path: '',
};
},
};
</script>
```
后端代码:
1. 展示HDFS文件列表
```
@RequestMapping("/list")
@ResponseBody
public List<FileStatus> listFiles() throws IOException {
FileSystem fs = FileSystem.get(new Configuration());
FileStatus[] fileStatuses = fs.listStatus(new Path("/"));
return Arrays.asList(fileStatuses);
}
```
2. 上传文件
```
@PostMapping("/upload")
@ResponseBody
public String uploadFile(MultipartFile file) throws IOException {
FileSystem fs = FileSystem.get(new Configuration());
Path dstPath = new Path(file.getOriginalFilename());
FSDataOutputStream outputStream = fs.create(dstPath);
IOUtils.copy(file.getInputStream(), outputStream);
outputStream.close();
fs.close();
return "上传成功";
}
```
3. 删除文件
```
@DeleteMapping("/delete")
@ResponseBody
public String deleteFile(String path) throws IOException {
FileSystem fs = FileSystem.get(new Configuration());
Path dstPath = new Path(path);
boolean isDeleted = fs.delete(dstPath, true);
fs.close();
return isDeleted ? "删除成功" : "删除失败";
}
```
4. 下载文件
```
@GetMapping("/download")
public ResponseEntity<byte[]> downloadFile(String path) throws IOException {
FileSystem fs = FileSystem.get(new Configuration());
Path dstPath = new Path(path);
FSDataInputStream inputStream = fs.open(dstPath);
byte[] fileBytes = IOUtils.toByteArray(inputStream);
inputStream.close();
fs.close();
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_OCTET_STREAM);
headers.setContentDispositionFormData("attachment", dstPath.getName());
return new ResponseEntity<>(fileBytes, headers, HttpStatus.OK);
}
```
相关推荐
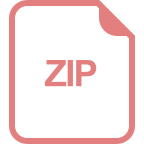
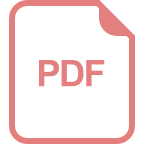




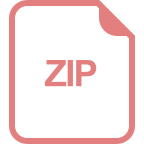
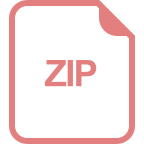
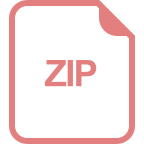
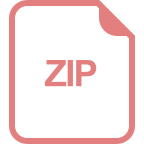
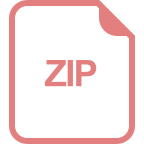