头歌python温度对照表
时间: 2024-12-17 10:52:03 浏览: 1
在Python中,我们可以创建一个简单的温度对照表,通常用来将摄氏度(Celsius)转换为华氏度(Fahrenheit)或者反过来。以下是一个基础的对照表:
- 摄氏度转华氏度的公式是: `F = C * 9/5 + 32`
- 华氏度转摄氏度的公式是: `C = (F - 32) * 5/9`
例如,你可以编写一个函数来实现这个转换:
```python
def celsius_to_fahrenheit(c):
return c * 9.0 / 5.0 + 32
def fahrenheit_to_celsius(f):
return (f - 32) * 5.0 / 9.0
```
然后你可以这样使用它们:
```python
# 转换示例
celsius_temp = 25
fahrenheit_temp = celsius_to_fahrenheit(celsius_temp)
print(f"{celsius_temp}℃等于{fahrenheit_temp}℉")
fahrenheit_temp = 77
celsius_temp = fahrenheit_to_celsius(fahrenheit_temp)
print(f"{fahrenheit_temp}℉等于{celsius_temp}℃")
```
相关问题
温度对照表Python头歌
温度对照表在Python中通常是通过字典或者列表数据结构来实现的,它将温度值映射到相应的描述或刻度上。比如常见的华氏(Fahrenheit)和摄氏(Celsius)之间的转换,可以创建一个这样的对照表:
```python
# 示例的温度对照表(华氏转摄氏)
temperature_conversion = {
"freezing": 0,
"cold": 32,
"cool": 50,
"average": 68,
"warm": 86,
"hot": 104,
"boiling": 212,
}
# 或者是简单的列表形式(例如只关心摄氏)
celsius_temps = [0, 10, 20, 30, 40, 50]
def fahrenheit_to_celsius(f):
return (f - 32) * 5 / 9
def celsius_to_fahrenheit(c):
return c * 9 / 5 + 32
```
你可以根据需要自定义这个对照表,并编写函数来进行转换。同时,Python的`str.format()`方法也可以方便地将温度值和对应的描述结合在一起。
用Python实现通过输入指定温度对照表的范围和温度值间隔,生成摄氏与华氏温度的对照表。从摄氏温度C到华氏温度F的计算公式是:F = C ×9/5+32
当然可以。首先,我们需要定义一个函数,该函数接受三个参数:最低摄氏温度(Celsius_min)、最高摄氏温度(Celsius_max)以及两个温度之间的步长(step)。然后,我们可以遍历这个温度区间,对于每个摄氏温度,我们使用给定的公式将其转换为华氏温度。以下是详细的Python代码实现:
```python
def celsius_to_fahrenheit(celsius_min, celsius_max, step):
# 定义转换函数
def celsius_to_fahrenheit_converter(celsius):
return (celsius * 9/5) + 32
# 创建一个空列表来存储结果
temperature_conversion_table = []
# 从最低摄氏温度开始,每次增加步长,直到达到或超过最高摄氏温度
for celsius in range(celsius_min, celsius_max+step, step):
fahrenheit = celsius_to_fahrenheit_converter(celsius)
temperature_conversion_table.append((celsius, fahrenheit))
# 返回包含所有转换的元组列表
return temperature_conversion_table
# 示例用法
min_temp_celsius = float(input("请输入最低摄氏温度: "))
max_temp_celsius = float(input("请输入最高摄氏温度: "))
temp_interval = float(input("请输入温度值间隔: "))
conversion_table = celsius_to_fahrenheit(min_temp_celsius, max_temp_celsius, temp_interval)
print("摄氏度到华氏度的对照表:")
for item in conversion_table:
print(f"{item[0]:.1f}°C = {item[1]:.1f}°F")
```
当你运行这段代码时,程序会提示你输入最低、最高摄氏温度和温度值间隔,然后会打印出对应范围内每增加一步温度值的摄氏度和华氏度转换结果。
阅读全文
相关推荐
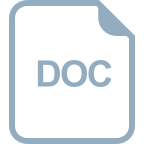
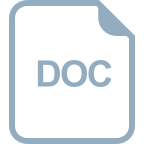
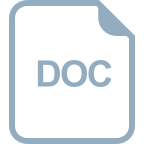
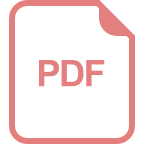
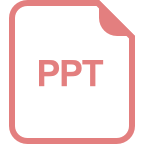
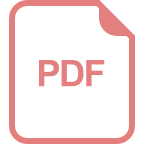
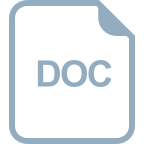
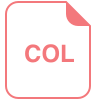
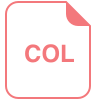







