c语言编写代码,完成: 1、创建一个带头结点的单链表,随机输入若干整数。 2、查找 结点数值等于dat的结点最大位序。(dat 需要外部输入) 3、插入 元素 到 指定 位置 ,其中元素值和位置都外部输入。 4、删除链表中的最大值。
时间: 2024-10-08 12:08:22 浏览: 53
在C语言中,我们可以使用结构体和指针来实现一个简单的单链表操作。以下是一个基本的例子,展示了如何完成上述四个任务:
1. 创建带头结点的单链表:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
void createList(int arr[], int size) {
Node* head = (Node*)malloc(sizeof(Node));
head->data = arr[0];
head->next = NULL;
for (int i = 1; i < size; i++) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = arr[i];
newNode->next = head->next;
head->next = newNode;
}
}
// 示例:arr[] 包含随机输入的整数数组
void inputArray(int arr[], int size) {
// ... 你可以添加代码从用户那里获取输入并填充数组
}
```
2. 查找等于dat的最大位序结点:
```c
Node* findMaxByOrder(Node* head, int dat) {
if (head == NULL || head->data > dat) return head;
return findMaxByOrder(head->next, dat);
}
```
3. 插入元素到指定位置:
```c
void insertElement(Node** head, int value, int position) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
if (*head == NULL || position == 0) {
newNode->next = *head;
*head = newNode;
return;
}
Node* current = *head;
for (int i = 1; i < position && current != NULL; i++)
current = current->next;
newNode->next = current->next;
current->next = newNode;
}
```
4. 删除链表中的最大值:
```c
void deleteMax(Node** head) {
if (*head == NULL) return;
Node* maxNode = *head;
Node* current = *head->next;
while (current != NULL && current->data > maxNode->data)
maxNode = current;
if (maxNode == *head)
*head = current;
else
maxNode->next = current->next;
free(current); // 释放内存
}
```
记得在实际使用前处理好用户输入,并在适当的地方添加错误检查。
阅读全文
相关推荐
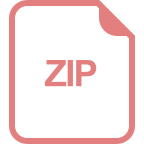
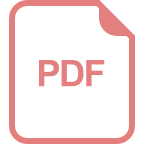
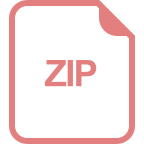















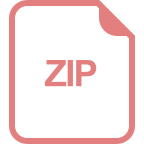