编写c语言程序,建立2个带头结点单链表,输入若干整数将正整数插入第1个单链表,将负整数插入第2个单链表,插入前和插入后单链表保持递增或相等次序,显示2个单链表,最后销毁。程序不可存在内存泄漏。
时间: 2024-03-23 18:16:11 浏览: 89
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Node{
int data;
struct Node* next;
}Node;
void insert(Node* head, int data){
Node* p = head;
while(p->next && p->next->data < data){
p = p->next;
}
Node* new_node = (Node*)malloc(sizeof(Node));
new_node->data = data;
new_node->next = p->next;
p->next = new_node;
}
void print_list(Node* head){
Node* p = head->next;
while(p){
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
void destroy_list(Node* head){
Node* p = head;
while(p){
Node* q = p;
p = p->next;
free(q);
}
}
int main(){
Node* head1 = (Node*)malloc(sizeof(Node));
head1->next = NULL;
Node* head2 = (Node*)malloc(sizeof(Node));
head2->next = NULL;
int n;
while(scanf("%d", &n) != EOF){
if(n > 0){
insert(head1, n);
}
else if(n < 0){
insert(head2, n);
}
}
print_list(head1);
print_list(head2);
destroy_list(head1);
destroy_list(head2);
return 0;
}
```
阅读全文
相关推荐




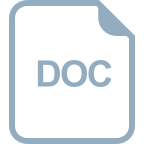


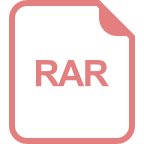
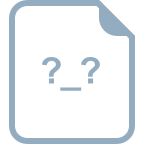
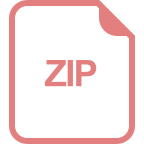