已知带头结点的双向循环链表头结点指针为list,除头结点外的每个链结点数据域值为一个整数,请写一个算法,将链表中所有数据域值大于0的结点放在所有数据域值小于0的结点之前。若链表中除头结点外没有其他结点,算法返回0,否则,算法返回1。写c语言代码
时间: 2024-11-27 20:16:47 浏览: 20
首先,我们需要明确的是,这是一个典型的链表操作问题,我们可以使用迭代的方式来解决,因为双向链表的特点使得我们可以在遍历的过程中同时访问前驱和后继节点。下面是一个简单的C语言代码实现:
```c
#include <stdio.h>
#include <stdbool.h>
// 定义双向链表节点结构体
typedef struct Node {
int data;
struct Node* prev; // 前驱指针
struct Node* next; // 后继指针
} Node;
// 插入节点到链表中间
void insert(Node** head, int data, bool isNegative) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->prev = NULL;
newNode->next = *head;
if (*head != NULL && isNegative) {
newNode->next->prev = newNode;
}
if (*head == NULL || !isNegative) {
*head = newNode;
} else {
while ((*head)->next->data < 0) {
head = &(*head)->next;
}
newNode->prev = *head->prev;
*head->prev->next = newNode;
newNode->prev->prev = newNode;
}
}
// 主函数
int rearrangeList(Node* list) {
if (list == NULL || list->next == list) { // 检查链表是否为空或者只有一个元素
return 0;
}
Node* current = list;
bool hasPositive = false, hasNegative = false;
// 遍历链表,标记正负数节点
while (current != NULL) {
if (current->data > 0) {
hasPositive = true;
break;
} else if (current->data < 0) {
hasNegative = true;
}
current = current->next;
}
// 如果既有正数又有负数,进行调整
if (hasPositive && hasNegative) {
insert(&list, -1, true); // 首先插入一个负一作为分界点
current = list;
while (current->next != list) {
if (current->data > 0) {
insert(¤t->next, current->data, false);
}
current = current->next;
}
return 1;
} else {
return 0;
}
}
// 打印链表
void printList(Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
int main() {
// 创建示例链表
Node* list = (Node*)malloc(sizeof(Node));
list->data = -5;
list->prev = NULL;
list->next = (Node*)malloc(sizeof(Node));
list->next->data = 0;
list->next->prev = list;
list->next->next = (Node*)malloc(sizeof(Node));
list->next->next->data = 4;
list->next->next->prev = list->next;
int result = rearrangeList(list);
if (result) {
printf("Rearranged List: ");
printList(list);
} else {
printf("No rearrangement needed.");
}
return 0;
}
```
阅读全文
相关推荐





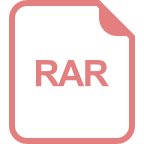








