用C++建立两个带头结点的单链表。输入若干整数,将正整数插入第一个单链表,将负整数插入第二个单链表。在插入前和插入后,两个单链表都要保持递增或相等的次序。最后,显示两个单链表,并销毁它们。在编写程序时,需要注意避免内存泄漏的问题。
时间: 2023-05-16 14:06:08 浏览: 74
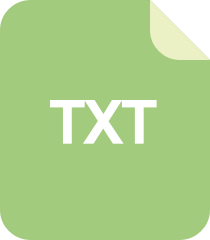
输入一个正整数,判断是不是素数(C++)
以下是用C语言实现的代码:
#include <stdio.h>
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
Node* createList() {
Node* head = (Node*)malloc(sizeof(Node));
head->next = NULL;
return head;
}
void insert(Node* head, int data) {
Node* p = head;
while (p->next != NULL && p->next->data < data) {
p = p->next;
}
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = p->next;
p->next = newNode;
}
void printList(Node* head) {
Node* p = head->next;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
}
void destroyList(Node* head) {
Node* p = head;
while (p != NULL) {
Node* q = p->next;
free(p);
p = q;
}
}
int main() {
Node* positiveList = createList();
Node* negativeList = createList();
int n;
while (scanf("%d", &n) != EOF) {
if (n > 0) {
insert(positiveList, n);
} else if (n < 0) {
insert(negativeList, n);
}
}
printList(positiveList);
printList(negativeList);
destroyList(positiveList);
destroyList(negativeList);
return 0;
}
阅读全文
相关推荐
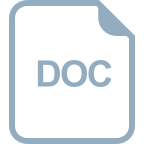
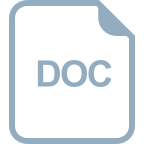




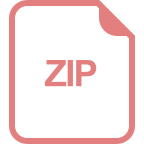
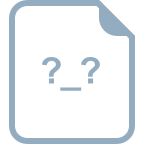
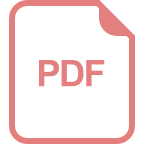

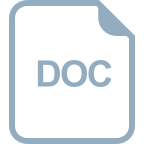
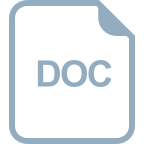
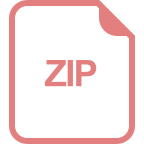