# 添加LSTM层 model.add(LSTM(4, return_sequences=True)) # 添加注意力机制. model.add(Attention()) model.add(Dense(2))这段程序什莫意思
时间: 2024-01-20 18:30:36 浏览: 31
这段程序是一个使用注意力机制的LSTM模型的示例代码。
首先,通过 `model.add(LSTM(4, return_sequences=True))` 添加一个具有4个隐藏单元的LSTM层,并设置 `return_sequences=True`,表示该LSTM层将返回完整的输出序列而不仅仅是最后一个时间步的输出。
然后,通过 `model.add(Attention())` 添加一个注意力层,用于引入注意力机制。这个注意力层将根据输入序列的相关性自动学习并加权考虑不同位置的信息。
最后,通过 `model.add(Dense(2))` 添加一个全连接层(Dense层),将注意力层的输出映射到两个输出节点,用于进行最终的分类或预测。
需要注意的是,上述代码只是一个示例,并不是完整的模型实现。具体的模型结构和参数设置可能会有所不同,取决于具体的问题和数据。此处提供的代码片段仅用于展示如何在模型中添加LSTM层、注意力层和全连接层。
相关问题
def create_LSTM_model(): # instantiate the model model = Sequential() model.add(Input(shape=(X_train.shape[1], X_train.shape[2]))) model.add(Reshape((X_train.shape[1], 1, X_train.shape[2], 1))) # cnn1d Layers model.add(ConvLSTM2D(filters=64, kernel_size=(1,3), activation='relu', padding='same', return_sequences=True)) model.add(Flatten()) model.add(Dropout(0.5)) # 添加lstm层 model.add(LSTM(64, activation = 'relu', return_sequences=True)) model.add(Dropout(0.5)) #添加注意力层 model.add(LSTM(64, activation = 'relu', return_sequences=False)) # 添加dropout model.add(Dropout(0.5)) model.add(Dense(128)) # 输出层 model.add(Dense(1, name='Output')) # 编译模型 model.compile(optimizer='adam', loss='mse', metrics=['mae']) return model # lstm network model = create_LSTM_model() # summary print(model.summary())修改该代码,解决ValueError: Input 0 of layer "lstm_22" is incompatible with the layer: expected ndim=3, found ndim=2. Full shape received: (None, 640)问题
根据错误信息,我们可以看出 "lstm_22" 层的输入维度(ndim)期望是3,但是实际上却是2。这个问题通常是由于输入数据形状(shape)不正确导致的。
在这个问题的代码中,我们可以看到在模型的第一层中添加了一个 Reshape 层,将输入数据的形状更改为 (batch_size, time_steps, features, 1, 1)。这是为了使其可以与 ConvLSTM2D 层兼容。
然而,在后续的层中,我们需要将数据形状转换回 (batch_size, time_steps, features)。因此,我们需要在 Reshape 层之后添加一个 Reshape 层,将数据形状从 (batch_size, time_steps, features, 1, 1) 转换为 (batch_size, time_steps, features)。
下面是修改过后的代码:
```python
def create_LSTM_model():
# instantiate the model
model = Sequential()
# add input layer
model.add(Input(shape=(X_train.shape[1], X_train.shape[2])))
# add reshape layer
model.add(Reshape((X_train.shape[1], 1, X_train.shape[2], 1)))
# cnn1d Layers
model.add(ConvLSTM2D(filters=64, kernel_size=(1,3), activation='relu', padding='same', return_sequences=True))
model.add(Flatten())
model.add(Dropout(0.5))
# add reshape layer
model.add(Reshape((X_train.shape[1], 64)))
# add lstm layer
model.add(LSTM(64, activation='relu', return_sequences=True))
model.add(Dropout(0.5))
# add attention layer
model.add(LSTM(64, activation='relu', return_sequences=False))
# add dropout layer
model.add(Dropout(0.5))
# add dense layers
model.add(Dense(128))
model.add(Dense(1, name='Output'))
# compile the model
model.compile(optimizer='adam', loss='mse', metrics=['mae'])
return model
# lstm network model
model = create_LSTM_model()
# summary
print(model.summary())
```
在上面的修改后的代码中,我们在 Reshape 层之后添加了一个新的 Reshape 层,将数据形状转换回 (batch_size, time_steps, features)。这样可以确保后续的 LSTM 层能够正确处理输入数据。
另外,我们还将 LSTM 层的 return_sequences 参数设置为 True,以确保输出的形状与输入形状相同。这样可以使得后续的层能够正确地处理输出数据。
# 定义模型 model = Sequential() # 添加CNN层 model.add( TimeDistributed(Conv1D(filters=64, kernel_size=1, activation='relu', input_shape=(None, 2, 1)))) model.add(TimeDistributed(MaxPooling1D(pool_size=1))) model.add(TimeDistributed(Flatten())) # 添加LSTM层 model.add(LSTM(4, return_sequences=True)) # 添加注意力机制 model.add(Attention()) model.add(Dense(2))
这是一个使用注意力机制的CNN+LSTM预测模型的定义,其中model是一个Sequential模型对象。Sequential模型是一种按顺序构建的模型,可以逐层添加神经网络层。
在这个模型中,首先使用了一个卷积神经网络(CNN)层,该层被TimeDistributed包裹,可以处理时间序列数据。在这里,我们使用了Conv1D层来进行一维卷积操作,并使用了MaxPooling1D层进行池化操作。
接着,在CNN层后面添加了一个Flatten层,将卷积层的输出展平。
然后,我们添加了一个长短期记忆网络(LSTM)层,该层的return_sequences参数设置为True,表示返回完整的序列输出而不只是最后一个时间步的输出。
最后,为了引入注意力机制,你可以在LSTM层之后添加一个注意力层,该层可以自动学习并加权考虑输入序列的不同部分。
最后,我们添加了一个Dense层来输出预测结果。
你可以根据自己的需求对模型进行调整和配置,例如添加更多的CNN或LSTM层,或者修改注意力层的结构。记得在使用模型之前,还需要进行编译(compile)和训练(fit)等步骤。
相关推荐
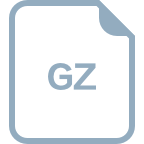
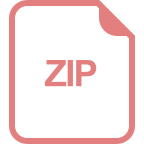








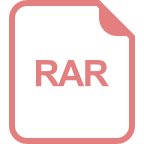