单链表的各种操作用C语言写出代码
时间: 2024-11-20 11:43:19 浏览: 19
单链表是一种基础的数据结构,它由节点组成,每个节点包含数据和指向下一个节点的指针。以下是单链表的一些基本操作,使用C语言进行编写:
1. 创建链表头结点(初始化一个空链表):
```c
typedef struct ListNode {
int data;
struct ListNode* next;
} ListNode;
ListNode* createList() {
return (ListNode*)malloc(sizeof(ListNode));
}
```
2. 插入节点到链表头部:
```c
void insertAtBegin(ListNode** head, int value) {
ListNode* newNode = createList();
newNode->data = value;
newNode->next = *head;
*head = newNode;
}
```
3. 插入节点到链表指定位置(假设链表已排序,通过遍历找到插入位置):
```c
void insertAtPosition(ListNode** head, int value, int position) {
if (*head == NULL || position <= 0) return;
ListNode* newNode = createList();
newNode->data = value;
ListNode* current = *head;
for (int i = 0; i < position - 1 && current != NULL; i++) {
current = current->next;
}
newNode->next = current;
if (current != NULL) current->next = newNode;
else *head = newNode;
}
```
4. 删除节点(删除给定值的第一个节点):
```c
void deleteNode(ListNode** head, int value) {
if (*head == NULL) return;
if ((*head)->data == value) {
ListNode* temp = *head;
*head = (*head)->next;
free(temp);
return;
}
ListNode* current = *head;
while (current->next != NULL && current->next->data != value) {
current = current->next;
}
if (current->next != NULL) {
ListNode* temp = current->next;
current->next = current->next->next;
free(temp);
}
}
```
5. 遍历链表并打印节点值:
```c
void printList(ListNode* head) {
ListNode* temp = head;
while (temp != NULL) {
printf("%d ", temp->data);
temp = temp->next;
}
printf("\n");
}
```
阅读全文
相关推荐
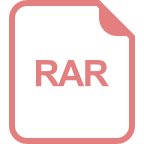
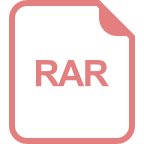
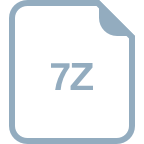















