走迷宫无墙壁队列C语言
时间: 2024-12-18 12:33:55 浏览: 3
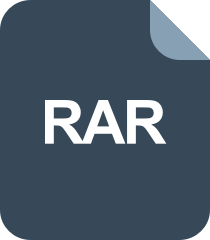
C语言走迷宫游戏源码和程序说明.rar
走迷宫无墙壁算法,也称为A*(A star)搜索算法,是一种启发式搜索算法,常用于解决寻路问题,如二维迷宫中的路径寻找。在C语言中实现A*算法,通常涉及以下几个步骤:
1. 定义数据结构:创建一个节点结构体,存储位置坐标、移动代价(g值)、预计代价(h值)和父节点指针。
```c
typedef struct Node {
int x, y; // 节点位置
int g, h, f; // g=已行走的距离, h=目标距离估计, f=g+h
struct Node *parent; // 父节点指针
} Node;
```
2. 初始化:设置起始节点和终止节点,并将起始节点标记为开集(open list),其他节点作为闭集(closed list)。
3. 搜索循环:直到找到终点或者开放列表为空。
- 从开放列表中选取f值最小的节点(即最有可能到达目标的节点)。
- 如果到达终点,回溯路径;否则,考虑该节点的所有邻居。
- 计算每个邻居的f值,如果邻居不在闭集中,加入到开放列表并更新其信息(包括g值和h值)。
- 否则,检查是否通过更短路径到达该邻居,如果是,则更新路径信息。
4. 回溯生成路径:从终点开始,通过`parent`指针依次获取所有节点,形成从起点到终点的路径。
C语言实现会涉及到优先队列(通常使用堆或斐波那契堆)来高效地处理搜索过程。以下是简化版的伪代码示例:
```c
void a_star_search(Node** maze_map, int start_x, int start_y, int end_x, int end_y) {
Node* open_list = create_heap();
Node* closed_list = create_list();
Node* start_node = create_node(start_x, start_y, 0, heuristic(start_x, start_y, end_x, end_y), NULL);
add_to_open(open_list, start_node);
while (!is_empty(open_list)) {
Node* current_node = get_min(open_list); // 获取f值最小的节点
if (current_node->x == end_x && current_node->y == end_y) {
build_path(current_node);
break;
}
remove_from_open(open_list, current_node);
add_to_closed(closed_list, current_node);
for (int i = 0; i < num_neighbors(current_node->x, current_node->y, maze_map); ++i) {
Node* neighbor = get_neighbor(i, current_node);
if (not_in(closed_list, neighbor)) {
update_costs(neighbor, current_node);
add_to_open(open_list, neighbor);
}
}
}
}
```
阅读全文
相关推荐
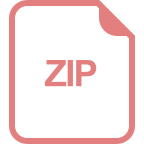
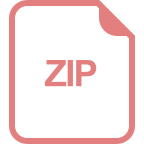













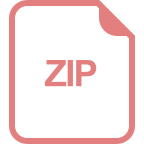
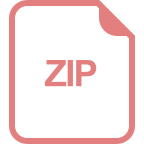
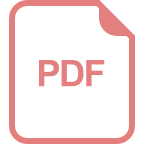