帮我写一个能玩的贪吃蛇的代码
时间: 2024-10-18 10:22:26 浏览: 21
当然可以!编写一个简单的贪吃蛇游戏的基本步骤包括创建游戏窗口、定义游戏角色(蛇)、食物生成、碰撞检测以及得分管理等。这里我会给你提供一个基础版本的Python游戏框架,使用`pygame`库来创建游戏。首先确保已经安装了`pygame`,如果没有,请运行`pip install pygame`。
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置窗口大小
window_width = 600
window_height = 400
screen = pygame.display.set_mode((window_width, window_height))
pygame.display.set_caption("贪吃蛇")
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
# 蛇的初始设置
snake_speed = 15
snake_pos = [(window_width // 2, window_height // 2)]
snake_body = [[window_width // 2, window_height // 2]]
direction = "right"
# 食物的初始位置
food_pos = [random.randint(1, window_width - 2), random.randint(1, window_height - 2)]
clock = pygame.time.Clock()
score = 0
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP and direction != "down":
direction = "up"
elif event.key == pygame.K_DOWN and direction != "up":
direction = "down"
elif event.key == pygame.K_LEFT and direction != "right":
direction = "left"
elif event.key == pygame.K_RIGHT and direction != "left":
direction = "right"
# 移动蛇并检测边界和碰撞
new_head = snake_pos[-1]
if direction == "up":
new_head = (new_head[0], new_head[1] - snake_speed)
elif direction == "down":
new_head = (new_head[0], new_head[1] + snake_speed)
elif direction == "left":
new_head = (new_head[0] - snake_speed, new_head[1])
else:
new_head = (new_head[0] + snake_speed, new_head[1])
snake_pos.insert(0, new_head)
# 检测蛇是否吃到食物
if snake_pos[0] == food_pos:
score += 10
# 生成新的食物位置
food_pos = [random.randint(1, window_width - 2), random.randint(1, window_height - 2)]
while food_pos in snake_body:
food_pos = [random.randint(1, window_width - 2), random.randint(1, window_height - 2)]
else:
tail = snake_body.pop()
screen.fill(BLACK)
# 绘制蛇的身体和头部
for pos in snake_body:
pygame.draw.rect(screen, WHITE, pygame.Rect(pos[0], pos[1], 10, 10))
pygame.draw.rect(screen, WHITE, pygame.Rect(new_head[0], new_head[1], 10, 10))
# 绘制食物
pygame.draw.rect(screen, WHITE, pygame.Rect(food_pos[0], food_pos[1], 10, 10))
# 更新屏幕
screen.blit(pygame.font.SysFont(None, 30).render(f"Score: {score}", 1, WHITE), (10, 10))
pygame.display.flip()
clock.tick(snake_speed)
```
这个代码实现了基本的贪吃蛇游戏,但没有处理蛇头碰撞身体的情况。要完成一个完整的游戏,你需要添加这些逻辑,例如当蛇头碰到墙壁或自己的身体时,游戏结束。
阅读全文
相关推荐
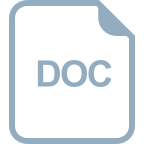
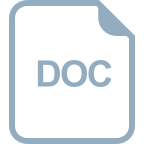
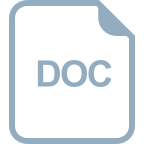
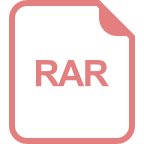
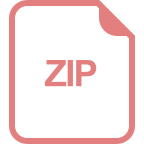
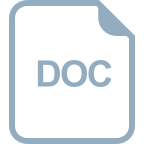
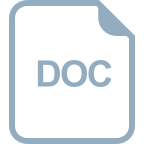
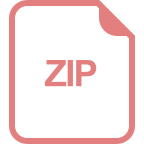
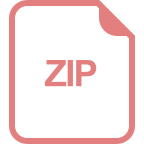
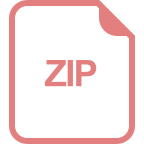
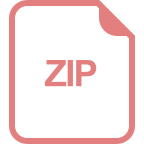
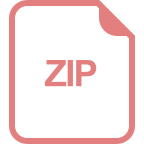
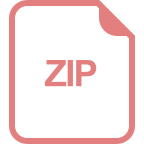
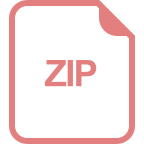
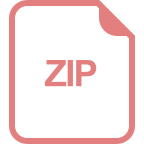