c语言:校园导航系统
时间: 2024-02-26 16:49:25 浏览: 119
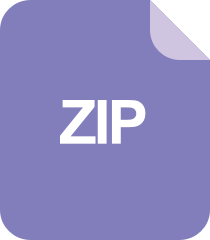
校园导航系统 c语言

校园导航系统是一个基于某学校地图的程序,它可以帮助用户找到从当前位置到校园其他位置的最短路径。该系统需要读者自己进行一些景点介绍和校园介绍的存储路径的更改,以确保功能的成功实现。
设计题目8中的校园导航系统也是类似的,它提供了校园各主要建筑的名称信息和有路线连通的信息,用户可以通过该系统找到从当前位置到目标位置的最短路径。
以下是一个C语言实现的校园导航系统的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTICES 100
#define INF 1000000
typedef struct {
int weight;
int from;
int to;
} Edge;
typedef struct {
int numVertices;
int numEdges;
int adjacencyMatrix[MAX_VERTICES][MAX_VERTICES];
} Graph;
void initializeGraph(Graph* graph, int numVertices) {
graph->numVertices = numVertices;
graph->numEdges = 0;
for (int i = 0; i < numVertices; i++) {
for (int j = 0; j < numVertices; j++) {
graph->adjacencyMatrix[i][j] = INF;
}
}
}
void addEdge(Graph* graph, int from, int to, int weight) {
graph->adjacencyMatrix[from][to] = weight;
graph->adjacencyMatrix[to][from] = weight;
graph->numEdges++;
}
void dijkstra(Graph* graph, int startVertex, int* distance, int* previous) {
int visited[MAX_VERTICES] = {0};
for (int i = 0; i < graph->numVertices; i++) {
distance[i] = INF;
previous[i] = -1;
}
distance[startVertex] = 0;
for (int i = 0; i < graph->numVertices; i++) {
int minDistance = INF;
int currentVertex = -1;
for (int j = 0; j < graph->numVertices; j++) {
if (!visited[j] && distance[j] < minDistance) {
minDistance = distance[j];
currentVertex = j;
}
}
if (currentVertex == -1) {
break;
}
visited[currentVertex] = 1;
for (int j = 0; j < graph->numVertices; j++) {
if (!visited[j] && graph->adjacencyMatrix[currentVertex][j] != INF) {
int newDistance = distance[currentVertex] + graph->adjacencyMatrix[currentVertex][j];
if (newDistance < distance[j]) {
distance[j] = newDistance;
previous[j] = currentVertex;
}
}
}
}
}
void printPath(Graph* graph, int* previous, int vertex) {
if (previous[vertex] != -1) {
printPath(graph, previous, previous[vertex]);
printf(" -> ");
}
printf("%d", vertex);
}
int main() {
Graph graph;
int numVertices = 6;
initializeGraph(&graph, numVertices);
addEdge(&graph, 0, 1, 7);
addEdge(&graph, 0, 2, 9);
addEdge(&graph, 0, 5, 14);
addEdge(&graph, 1, 2, 10);
addEdge(&graph, 1, 3, 15);
addEdge(&graph, 2, 3, 11);
addEdge(&graph, 2, 5, 2);
addEdge(&graph, 3, 4, 6);
addEdge(&graph, 4, 5, 9);
int startVertex = 0;
int distance[MAX_VERTICES];
int previous[MAX_VERTICES];
dijkstra(&graph, startVertex, distance, previous);
for (int i = 0; i < numVertices; i++) {
printf("Shortest path from %d to %d: ", startVertex, i);
printPath(&graph, previous, i);
printf("\n");
}
return 0;
}
```
该示例代码使用了Dijkstra算法来计算最短路径。首先,我们需要定义一个Graph结构体来表示图,其中包括顶点数和边数以及邻接矩阵。然后,我们可以使用initializeGraph函数初始化图,并使用addEdge函数添加边。接下来,使用dijkstra函数计算从起始顶点到其他顶点的最短路径,并将结果存储在distance和previous数组中。最后,使用printPath函数打印出从起始顶点到每个顶点的最短路径。
请注意,这只是一个简单的示例代码,实际的校园导航系统可能需要更复杂的数据结构和算法来处理实际的地图数据。
阅读全文
相关推荐
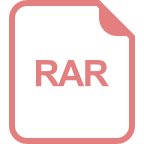
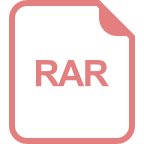
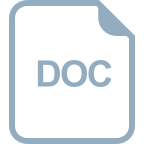
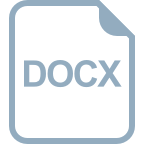
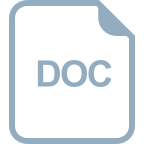
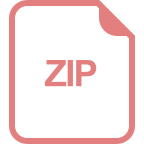
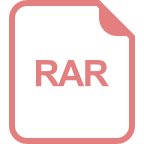
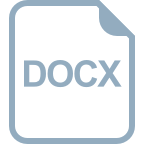
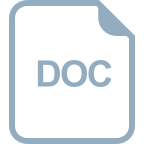
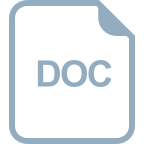
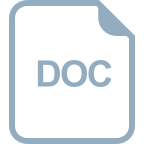




