python中小猫咪动态
时间: 2024-09-29 08:01:53 浏览: 17
在Python中,"小猫咪动态"通常是指使用Python的类(Class)和对象(Object)的概念来模拟猫咪的行为。你可以创建一个名为`Cat`的类,包含猫咪的一些属性(如颜色、名字)和方法(如叫声、吃食)。例如:
```python
class Cat:
def __init__(self, name, color):
self.name = name
self.color = color
def meow(self):
print(f"{self.name} says 'Meow!'")
def eat(self, food):
print(f"{self.name} is eating {food}")
# 创建一只小猫咪实例
kitty = Cat("花花", "白色")
kitty.meow() # 输出:花花 says 'Meow!'
kitty.eat("鱼") # 输出:花花 is eating 鱼
```
这里的小猫咪可以根据不同的实例有不同的行为,这就是Python中面向对象编程的多态性体现。当你需要让程序处理多种类型的猫(比如波斯猫、暹罗猫),只需要创建不同的子类继承自`Cat`,并覆盖或添加新的方法即可。
相关问题
python画小猫咪代码
这里是一个使用Python绘制小猫咪的示例代码:
```python
import turtle
# 绘制猫脸
def draw_face():
turtle.pensize(5)
turtle.penup()
turtle.goto(0, -100)
turtle.pendown()
turtle.circle(100)
# 绘制猫耳朵
def draw_ears():
turtle.fillcolor("pink")
turtle.penup()
turtle.goto(-70, 70)
turtle.pendown()
turtle.begin_fill()
turtle.right(45)
turtle.forward(50)
turtle.right(135)
turtle.forward(100)
turtle.right(135)
turtle.forward(50)
turtle.end_fill()
turtle.fillcolor("pink")
turtle.penup()
turtle.goto(70, 70)
turtle.pendown()
turtle.begin_fill()
turtle.left(45)
turtle.forward(50)
turtle.left(135)
turtle.forward(100)
turtle.left(135)
turtle.forward(50)
turtle.end_fill()
# 绘制猫眼睛
def draw_eyes():
turtle.fillcolor("white")
turtle.penup()
turtle.goto(-40, 20)
turtle.pendown()
turtle.begin_fill()
turtle.circle(20)
turtle.end_fill()
turtle.fillcolor("black")
turtle.penup()
turtle.goto(-40, 25)
turtle.pendown()
turtle.begin_fill()
turtle.circle(10)
turtle.end_fill()
turtle.fillcolor("white")
turtle.penup()
turtle.goto(40, 20)
turtle.pendown()
turtle.begin_fill()
turtle.circle(20)
turtle.end_fill()
turtle.fillcolor("black")
turtle.penup()
turtle.goto(40, 25)
turtle.pendown()
turtle.begin_fill()
turtle.circle(10)
turtle.end_fill()
# 绘制猫嘴巴
def draw_mouth():
turtle.penup()
turtle.goto(-30, -20)
turtle.pendown()
turtle.right(45)
turtle.circle(30, 90)
# 绘制猫身体
def draw_body():
turtle.fillcolor("gray")
turtle.penup()
turtle.goto(0, -200)
turtle.pendown()
turtle.begin_fill()
turtle.circle(100)
turtle.end_fill()
# 绘制猫爪子
def draw_paws():
turtle.fillcolor("pink")
turtle.penup()
turtle.goto(-50, -150)
turtle.pendown()
turtle.begin_fill()
turtle.circle(20)
turtle.end_fill()
turtle.fillcolor("pink")
turtle.penup()
turtle.goto(50, -150)
turtle.pendown()
turtle.begin_fill()
turtle.circle(20)
turtle.end_fill()
# 绘制猫尾巴
def draw_tail():
turtle.penup()
turtle.goto(80, -200)
turtle.pendown()
turtle.right(45)
turtle.circle(50, 90)
# 主函数
def main():
turtle.speed(0)
draw_face()
draw_ears()
draw_eyes()
draw_mouth()
draw_body()
draw_paws()
draw_tail()
turtle.done()
if __name__ == "__main__":
main()
```
运行代码,就可以绘制出一个可爱的小猫咪了!
python做一个小猫咪动态
在Python中制作一个小猫咪动态,我们可以利用PIL(Pillow)库来创建简单的图像处理动画。下面是一个基本的例子,展示如何使用`ImageDraw`模块画出一只简化的动态小猫:
```python
import os
from PIL import Image, ImageDraw, ImageFont
# 定义小猫咪的形状
def draw_cat(x, y):
img = Image.new('RGB', (50, 50), color='white')
draw = ImageDraw.Draw(img)
draw.rectangle([(10, 10), (40, 40)], fill='black') # 眼睛
draw.rectangle([(15, 20), (35, 30)], fill='black') # 鼻子
draw.line([(15, 25), (25, 35)], fill='black') # 上唇线
draw.line([(25, 25), (15, 35)], fill='black') # 下唇线
img.paste(Image.open('cat_face.png'), (x, y)) # 小猫的脸部贴图
return img
# 创建一个空白画布
canvas = Image.new('RGB', (200, 200))
font = ImageFont.truetype("arial.ttf", size=24)
# 动态效果设置
delay = 100 # 每帧延迟时间(毫秒)
frames = 50 # 总帧数
for i in range(frames):
x = i * 30 # 小猫咪从左向右移动
cat = draw_cat(x, 75) # 画出小猫
canvas.paste(cat, (0, 0)) # 在画布上放置小猫
text = f"Frame {i+1}/{frames}"
canvas_draw = ImageDraw.Draw(canvas)
canvas_draw.text((10, 10), text, font=font, fill=(0, 0, 0))
# 保存每一帧
canvas.save(f"frame_{i}.png", 'PNG')
if i < frames - 1:
canvas.show() # 显示进度帧
time.sleep(delay / 1000.0)
print("Animation complete.")
相关推荐
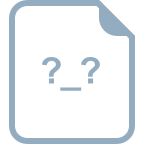
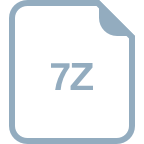
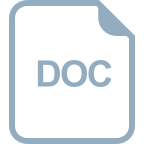


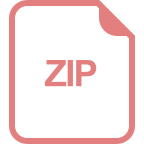
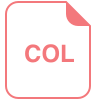







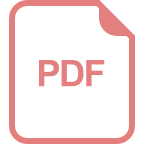