在pyqt5画布上存在多个图形对象,全选后,鼠标选中其中一个对象拖拽移动,要求,其他对象与选中对象在y轴上的距离越远,跟随鼠标移动时,y轴偏移量越小,x轴偏移量与鼠标偏移量相同。
时间: 2024-12-20 12:20:48 浏览: 4
在PyQt5中,实现这种效果通常需要结合QGraphicsScene和QGraphicsView组件,以及QGraphicsItem子类来操作图形对象。首先,你需要为每个图形对象创建一个自定义的QGraphicsItem子类,并存储它们在场景中的位置信息以及与其他对象的关系。
1. 创建图形项类:为每个图形对象创建一个继承自QGraphicsItem的类,比如`CustomGraphicsObject`,并维护它的位置属性(如x和y坐标),以及与其它对象的距离数据。
```python
class CustomGraphicsObject(QGraphicsItem):
def __init__(self, parent=None, distance=0):
super().__init__(parent)
self.pos = QPointF(0, 0) # 起始位置
self.distance_data = distance
def move_by(self, dx, dy):
new_y = self.y() + dy * (1 / abs(self.distance_data)) # 根据距离调整垂直偏移
self.setPos(self.x() + dx, new_y)
```
2. 设置场景和视图:在`QGraphicsScene`中添加所有`CustomGraphicsObject`实例,并设置`QGraphicsView`为场景的视口。
```python
scene = QGraphicsScene()
objects = [CustomGraphicsObject(distance=i) for i in range(len(objects))] # 假设objects是一个图形对象列表
for obj in objects:
scene.addItem(obj)
view = QGraphicsView(scene)
```
3. 鼠标事件处理:在`QGraphicsView`上连接鼠标的拖动事件,更新所选物体的位置并同步其他物体的位置变化。
```python
def mouseMoveEvent(event):
selected_object = scene.itemAt(event.scenePos()) # 获取当前被选中的对象
if selected_object is not None and isinstance(selected_object, CustomGraphicsObject):
selected_object.move_by(event.dx(), event.dy()) # 更新选中对象的位置
# 计算新位置下的偏移量,并同步其他物体
for other_obj in scene.items():
if other_obj != selected_object:
other_obj.move_by(event.dx(), event.dy() * (1 / selected_object.distance_data))
view.installEventFilter(self) # 添加事件过滤器处理鼠标事件
```
阅读全文
相关推荐



















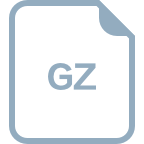