编写一个C++程序,给定一个整数数组 nums 和一个整数目标值 target,请你在该数组中找出 和为目标值 target 的那 两个 整数,并返回它们的数组下标。 你可以假设每种输入只会对应一个答案,并且你不能使用两次相同的元素。
时间: 2024-10-08 16:07:44 浏览: 33
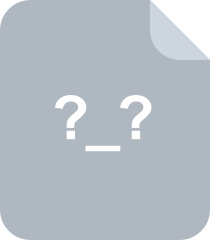
最接近的三数之和.md
在C++中,你可以使用哈希表(unordered_set)来解决这个问题。哈希表提供快速查找的功能,可以帮助我们在O(1)时间内找到目标值减去当前元素的差。以下是解决方案:
```cpp
#include <vector>
#include <unordered_set>
std::pair<int, int> twoSum(std::vector<int>& nums, int target) {
std::unordered_set<int> hashTable;
for (size_t i = 0; i < nums.size(); ++i) {
int complement = target - nums[i];
if (hashTable.find(complement) != hashTable.end()) {
return {hashTable[complement], i};
}
hashTable.insert(nums[i]);
}
throw std::runtime_error("No solution found"); // 如果没有找到解,抛出异常
}
int main() {
std::vector<int> nums = {2, 7, 11, 15}; // 示例数组
int target = 9; // 目标值
try {
std::pair<int, int> indices = twoSum(nums, target);
std::cout << "Indices of the two numbers that add up to " << target << ": "
<< indices.first << " and " << indices.second << std::endl;
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
这个程序首先遍历数组,对每个元素检查是否存在目标值减去它的补数的元素,如果存在,则返回这两个元素的索引。如果遍历结束还没有找到,就说明不存在这样的两个数,抛出异常。
阅读全文
相关推荐
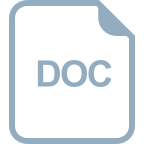
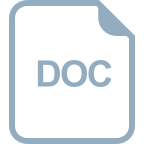












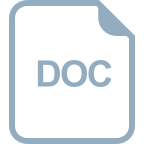
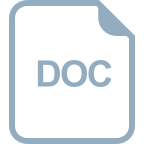
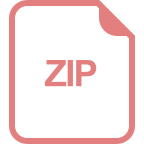
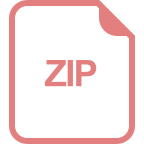