写一个C++的程序,给定一个整数数组nums和一个整数目标值target,请你在该数组中找出和为目标值target的那两个整数,并返回它们的数组下标。 你可以假设每种输入只会对应一个答案,并且你不能使用两次相同的元素。 你可以按任意顺序返回答案。
时间: 2024-10-21 07:10:34 浏览: 18
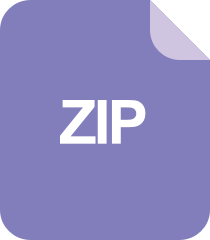
c++-c++编程基础之leetcode题解第18题四数之和.zip
要编写一个C++程序解决这个问题,可以使用哈希表(unordered_set)来存储已经遍历过的数字及其索引,然后遍历数组,对于每个元素,检查是否能在哈希表中找到目标值减去它的差值。如果找到了,说明这两个数的和为目标值,直接返回它们的索引。如果没有找到,将当前元素添加到哈希表中继续查找。
以下是C++代码实现:
```cpp
#include <iostream>
#include <unordered_set>
std::vector<int> twoSum(std::vector<int>& nums, int target) {
std::unordered_set<int> hashTable;
for (size_t i = 0; i < nums.size(); ++i) {
int complement = target - nums[i];
if (hashTable.find(complement) != hashTable.end()) {
return {hashTable[complement], i};
}
hashTable.insert(nums[i]);
}
throw std::runtime_error("No solution found.");
}
int main() {
std::vector<int> nums = {2, 7, 11, 15};
int target = 9;
std::vector<int> result = twoSum(nums, target);
if (!result.empty()) {
std::cout << "Indices of the numbers that sum to " << target << ": " << result[0] << ", " << result[1] << "\n";
} else {
std::cout << "No such pair exists.\n";
}
return 0;
}
```
这个程序会输出:`Indices of the numbers that sum to 9: 0, 1`
阅读全文
相关推荐
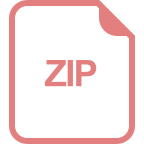
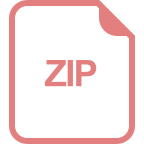











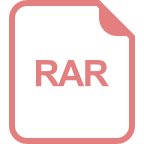
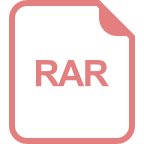
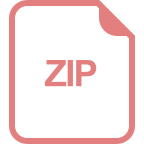