如何利用python写一个收银系统
时间: 2024-09-29 09:13:05 浏览: 53
编写一个简单的Python收银系统可以分为几个步骤,这里提供一个基础框架示例:
1. **项目初始化**:
首先,创建一个新的Python文件,如`checkout_system.py`,导入必要的模块,比如`os`、`datetime`用于基本操作。
```python
import os
from datetime import datetime
```
2. **商品类(Product)**:
定义一个商品类,包含名称、价格和库存属性。
```python
class Product:
def __init__(self, name, price, stock):
self.name = name
self.price = price
self.stock = stock
```
3. **购物车类(ShoppingCart)**:
添加一些方法,如添加商品、删除商品、查看购物车内容等。
```python
class ShoppingCart:
def __init__(self):
self.items = []
def add_item(self, product, quantity):
self.items.append((product, quantity))
def remove_item(self, item_name):
self.items = [(p, q) for p, q in self.items if p.name != item_name]
def display_cart(self):
total_cost = 0
print("Shopping Cart:")
for product, quantity in self.items:
total_cost += product.price * quantity
print(f"{product.name} x {quantity}: ${product.price*quantity}")
return total_cost
```
4. **主函数(main function)**:
用户交互部分,接收用户输入并调用购物车操作。
```python
def main():
products = [Product("Apple", 5, 10), Product("Banana", 3, 20)] # 示例商品列表
cart = ShoppingCart()
while True:
user_input = input("Enter 'add' to add an item, 'remove' to remove one, 'view' to see cart, or 'checkout' to finish: ")
if user_input == "add":
item_name = input("Item name: ")
if item_name in [p.name for p in products]:
quantity = int(input("Quantity: "))
cart.add_item(products[products.index(Product(item_name))], quantity)
elif user_input == "remove":
item_to_remove = input("Remove which item? ")
cart.remove_item(item_to_remove)
elif user_input == "view":
total = cart.display_cart()
print(f"Total cost: ${total}")
elif user_input == "checkout":
break
if __name__ == "__main__":
main()
```
这只是一个非常基础的框架,实际应用中可能需要处理更多细节,例如数据库存储商品信息、错误处理、支付功能等。运行此脚本后,用户可以通过命令行进行操作。
阅读全文
相关推荐
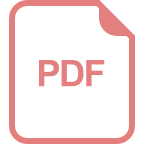
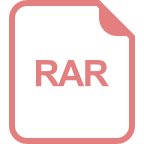
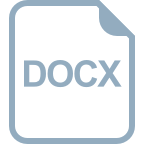
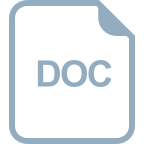
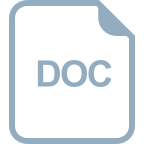












