基于Python的购物网页基于Python的购物网页代码
时间: 2024-09-12 15:10:10 浏览: 14
基于Python构建一个简单的购物网页通常会涉及到Web框架,如Django或Flask。这里我会给你一个基本的示例,展示如何使用Flask框架创建一个基本的购物车功能:
```python
from flask import Flask, render_template, request, redirect
app = Flask(__name__)
# 假设我们有一个商品列表存储在字典中
products = {
'product1': {'name': '产品A', 'price': 99},
'product2': {'name': '产品B', 'price': 149},
}
@app.route('/')
def index():
return render_template('index.html', products=products)
@app.route('/add_to_cart/<product_id>', methods=['POST'])
def add_to_cart(product_id):
# 这里只是一个简单的模拟,实际应用需要持久化储存购物车信息
if product_id in products:
# 假设购物车是一个全局变量,或者从数据库获取
cart = {'product1': 1, 'product2': 0} # 初始化空购物车
cart[product_id] = cart.get(product_id, 0) + 1
return redirect('/')
else:
return "商品不存在"
if __name__ == '__main__':
app.run(debug=True)
```
在这个例子中,`index`函数渲染主页面,显示所有商品。用户点击某个商品添加到购物车的操作通过`add_to_cart`路由处理,它接收POST请求并更新购物车。
对应的HTML模板`index.html`可能包括商品列表以及添加按钮:
```html
<!DOCTYPE html>
<html>
<head>
<title>购物网站</title>
</head>
<body>
{% for product in products %}
<h3>{{ product.name }} - {{ product.price }}</h3>
<form action="/add_to_cart/{{ product.id }}" method="post">
<button type="submit">加入购物车</button>
</form>
{% endfor %}
<!-- 模拟购物车区域 -->
<p>购物车: {{ cart|join(', ') }}</p>
</body>
</html>
```
这只是一个非常基础的示例,真实的购物网站会更复杂,涉及库存管理、支付处理、用户认证等更多功能。
相关推荐
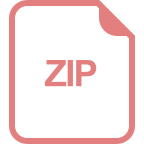
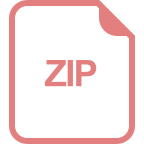
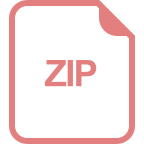














