vue 获取当前时间的时分秒
时间: 2023-11-10 20:02:08 浏览: 256
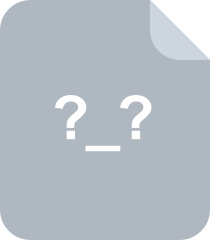
vue时间戳转年月日时分的过滤器
在Vue中,你可以使用JavaScript的Date对象来获取当前时间的时、分、秒。以下是一种实现方式:
```javascript
// 获取当前时间
let currentTime = new Date();
// 获取时分秒
let hours = currentTime.getHours();
let minutes = currentTime.getMinutes();
let seconds = currentTime.getSeconds();
// 将时分秒格式化为两位数
hours = String(hours).padStart(2, '0');
minutes = String(minutes).padStart(2, '0');
seconds = String(seconds).padStart(2, '0');
// 输出结果
console.log(`${hours}:${minutes}:${seconds}`);
```
阅读全文
相关推荐
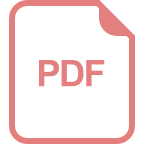















