numpy.core._exceptions._UFuncNoLoopError: ufunc 'add' did not contain a loop with signature matching types (dtype('<U1'), dtype('<U1')) -> None
时间: 2024-01-09 08:03:43 浏览: 64
这个错误通常是由于在使用NumPy的ufunc函数进行数组操作时,出现了类型不匹配的问题。具体来说,这个错误是因为ufunc函数 'add' 的输入类型不匹配造成的。
在你的代码中,可能出现了两个字符串类型(dtype('<U1'))的数组进行相加操作,而NumPy的ufunc函数需要相同类型的数组进行操作。请确保你传入 'add' 函数的两个数组具有相同的数据类型。
如果你希望将两个字符串数组进行逐元素相加的操作,你可以考虑使用字符串连接的方式,而不是直接使用NumPy的add函数。例如,可以使用字符串拼接操作符 '+' 来实现:
```python
result = array1 + array2
```
或者使用NumPy的vectorize函数将字符串拼接操作转换为ufunc函数:
```python
import numpy as np
# 定义一个字符串拼接函数
def concatenate_strings(a, b):
return a + b
# 将字符串拼接函数转换为ufunc函数
concatenate_strings_ufunc = np.vectorize(concatenate_strings)
# 使用ufunc函数进行字符串拼接
result = concatenate_strings_ufunc(array1, array2)
```
请根据你的实际需求选择适合的方法来处理这个错误。
相关推荐
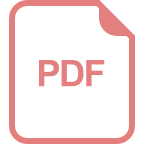
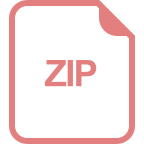
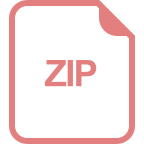
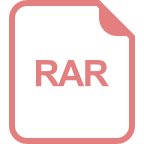
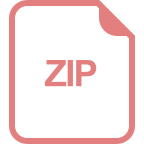
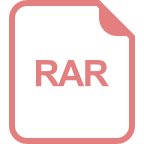
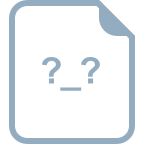
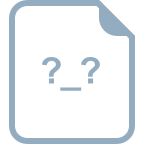
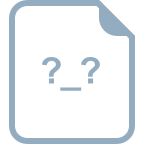