单链表的各种基本运算
时间: 2024-03-23 17:32:11 浏览: 30
单链表是一种常见的数据结构,它由一系列节点组成,每个节点包含一个数据元素和一个指向下一个节点的指针。单链表的基本运算包括:
1. 初始化:创建一个空链表。
2. 插入:在链表的指定位置插入一个新节点。
3. 删除:删除链表中指定位置的节点。
4. 查找:查找链表中指定位置的节点或者包含指定数据元素的节点。
5. 遍历:依次访问链表中的每个节点。
下面是单链表的基本操作实现:
```python
class Node:
def __init__(self, data):
self.data = data
self.next = None
class LinkedList:
def __init__(self):
self.head = None
# 在链表末尾添加新节点
def append(self, data):
new_node = Node(data)
if not self.head:
self.head = new_node
return
curr_node = self.head
while curr_node.next:
curr_node = curr_node.next
curr_node.next = new_node
# 在链表指定位置插入新节点
def insert(self, pos, data):
if pos < 0:
raise ValueError("Invalid position")
new_node = Node(data)
if pos == 0:
new_node.next = self.head
self.head = new_node
return
curr_pos = 0
curr_node = self.head
while curr_pos < pos - 1 and curr_node:
curr_node = curr_node.next
curr_pos += 1
if not curr_node:
raise ValueError("Invalid position")
new_node.next = curr_node.next
curr_node.next = new_node
# 删除链表指定位置的节点
def delete(self, pos):
if pos < 0:
raise ValueError("Invalid position")
if pos == 0:
self.head = self.head.next
return
curr_pos = 0
curr_node = self.head
while curr_pos < pos - 1 and curr_node:
curr_node = curr_node.next
curr_pos += 1
if not curr_node or not curr_node.next:
raise ValueError("Invalid position")
curr_node.next = curr_node.next.next
# 查找链表指定位置的节点
def get_node(self, pos):
if pos < 0:
raise ValueError("Invalid position")
curr_pos = 0
curr_node = self.head
while curr_pos < pos and curr_node:
curr_node = curr_node.next
curr_pos += 1
if not curr_node:
raise ValueError("Invalid position")
return curr_node
# 查找链表中包含指定数据元素的节点
def find(self, data):
curr_node = self.head
while curr_node and curr_node.data != data:
curr_node = curr_node.next
return curr_node
# 遍历链表并输出每个节点的数据元素
def traverse(self):
curr_node = self.head
while curr_node:
print(curr_node.data)
curr_node = curr_node.next
```
相关推荐
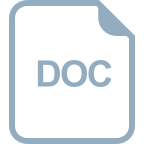














