设计整数单链表的基本运算程序,并用相关数据进行测试
时间: 2023-04-12 10:02:34 浏览: 269
好的,以下是整数单链表的基本运算程序:
1. 定义结构体Node,表示链表中的节点,包含一个整数值和一个指向下一个节点的指针。
```
struct Node {
int value;
Node* next;
};
```
2. 定义链表类LinkedList,包含头节点指针和链表长度。
```
class LinkedList {
public:
LinkedList();
~LinkedList();
void insert(int value); // 在链表末尾插入一个节点
void remove(int value); // 删除链表中第一个值为value的节点
void print(); // 打印链表中所有节点的值
int length(); // 返回链表长度
private:
Node* head;
int len;
};
```
3. 实现链表类的构造函数、析构函数和基本操作函数。
```
LinkedList::LinkedList() {
head = nullptr;
len = ;
}
LinkedList::~LinkedList() {
Node* p = head;
while (p != nullptr) {
Node* q = p->next;
delete p;
p = q;
}
}
void LinkedList::insert(int value) {
Node* p = new Node;
p->value = value;
p->next = nullptr;
if (head == nullptr) {
head = p;
} else {
Node* q = head;
while (q->next != nullptr) {
q = q->next;
}
q->next = p;
}
len++;
}
void LinkedList::remove(int value) {
if (head == nullptr) {
return;
}
if (head->value == value) {
Node* p = head;
head = head->next;
delete p;
len--;
return;
}
Node* p = head;
while (p->next != nullptr && p->next->value != value) {
p = p->next;
}
if (p->next != nullptr) {
Node* q = p->next;
p->next = q->next;
delete q;
len--;
}
}
void LinkedList::print() {
Node* p = head;
while (p != nullptr) {
cout << p->value << " ";
p = p->next;
}
cout << endl;
}
int LinkedList::length() {
return len;
}
```
4. 使用相关数据进行测试。
```
int main() {
LinkedList list;
list.insert(1);
list.insert(2);
list.insert(3);
list.print(); // 输出:1 2 3
list.remove(2);
list.print(); // 输出:1 3
cout << list.length() << endl; // 输出:2
return ;
}
```
阅读全文
相关推荐
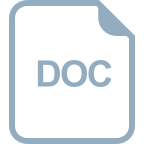
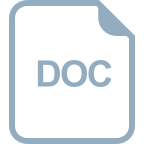
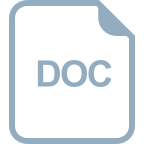






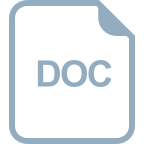
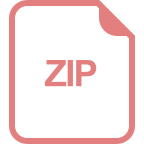
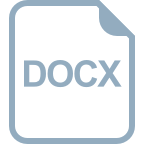
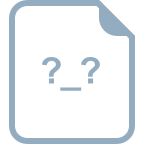
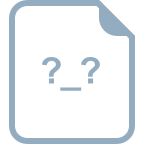
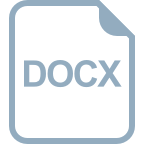
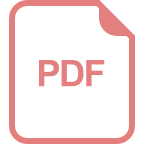
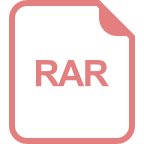
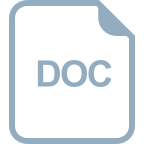
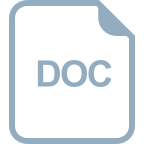