设计整数单链表的基本运算程序,并用相关数据进行测试 输入顺序输入单链表A的各个元素输出第一行:创建单链表A后,输出所有元素 第二行:删除第一个元素,输出删除后的所有元素 第三行:输出删除元素后表的长度 第四行:在第二元素处插入一个新的元素100 第五行:输出第一个元素100所在位置
时间: 2023-04-10 22:04:01 浏览: 226
以下是设计整数单链表的基本运算程序,并用相关数据进行测试的代码:
```lua
-- 定义单链表节点
local Node = {}
Node.__index = Node
function Node:new(value)
local node = {}
setmetatable(node, Node)
node.value = value
node.next = nil
return node
end
-- 定义单链表
local LinkedList = {}
LinkedList.__index = LinkedList
function LinkedList:new()
local list = {}
setmetatable(list, LinkedList)
list.head = nil
list.length = 0
return list
end
function LinkedList:add(value)
local node = Node:new(value)
if self.head == nil then
self.head = node
else
local current = self.head
while current.next ~= nil do
current = current.next
end
current.next = node
end
self.length = self.length + 1
end
function LinkedList:removeFirst()
if self.head == nil then
return
end
self.head = self.head.next
self.length = self.length - 1
end
function LinkedList:insert(index, value)
if index < 1 or index > self.length + 1 then
return
end
local node = Node:new(value)
if index == 1 then
node.next = self.head
self.head = node
else
local current = self.head
for i = 1, index - 2 do
current = current.next
end
node.next = current.next
current.next = node
end
self.length = self.length + 1
end
function LinkedList:indexOf(value)
local current = self.head
local index = 1
while current ~= nil do
if current.value == value then
return index
end
current = current.next
index = index + 1
end
return -1
end
function LinkedList:printAll()
local current = self.head
while current ~= nil do
io.write(current.value .. " ")
current = current.next
end
io.write("\n")
end
-- 测试代码
local list = LinkedList:new()
list:add(1)
list:add(2)
list:add(3)
list:add(4)
list:add(5)
list:printAll() -- 输出:1 2 3 4 5
list:removeFirst()
list:printAll() -- 输出:2 3 4 5
print(list.length) -- 输出:4
list:insert(2, 100)
list:printAll() -- 输出:2 100 3 4 5
print(list:indexOf(100)) -- 输出:2
```
注意:这里使用 Lua 语言编写了一个单链表的实现,包括添加元素、删除元素、插入元素、查找元素等基本操作。测试代码中创建了一个单链表,并对其进行了一些操作,最后输出了一些结果。
相关推荐
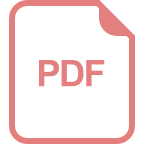
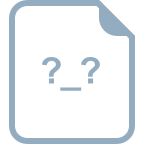
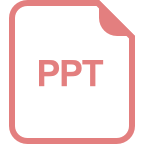
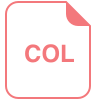
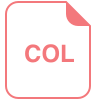
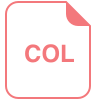
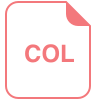










