C语言多线程适配libnuma样例
时间: 2024-08-28 11:03:09 浏览: 51
C语言中的多线程适配libnuma(Linux NUMA架构支持库)主要是为了优化内存访问性能,当应用程序运行在一个包含非均匀内存访问(Non-Uniform Memory Access, NUMA)节点的系统上时。NUMA设计下,处理器对近在咫尺的本地内存访问速度更快,而远距离的内存访问则较慢。
以下是一个简单的示例,展示如何使用libnuma在C语言中管理线程并分配给特定的 NUMA 节点:
```c
#include <stdio.h>
#include <pthread.h>
#include <numa.h>
// 获取当前进程的NUMA节点编号
static int get_my_node() {
return numa_node_id();
}
void* thread_func(void* arg) {
// 确保每个线程在启动时被绑定到合适的NUMA节点
numa_node_t node = *(numa_node_t*)arg;
if (node >= 0 && numa_bind pthread_self(), node, numa_node_membind) {
fprintf(stderr, "Error binding to node %d\n", node);
exit(1);
}
printf("Thread started on node %d\n", numa_node_id());
// 进行耗时操作...
// ...
return NULL;
}
int main() {
numa_nodeset_t nodeset;
numa_create_node_set(&nodeset, numa_num_nodes);
// 创建线程并绑定到每个NUMA节点
for (int i = 0; i < numa_num_nodes(); ++i) {
numa_add_node_to_set(&nodeset, i);
pthread_t tid;
pthread_attr_t attr;
pthread_attr_init(&attr);
pthread_attr_setinheritsched(&attr, PTHREAD_EXPLICIT_SCHED);
pthread_attr_setschedpolicy(&attr, SCHED_RR); // 使用实时调度策略
pthread_t *thread_ptr = malloc(sizeof(pthread_t));
*thread_ptr = pthread_create(thread_ptr, &attr, thread_func, (void*)&i);
if (thread_ptr == NULL) {
perror("Creating thread");
exit(1);
}
}
// 等待所有线程完成
pthread_join(*thread_ptr, NULL);
numa_destroy_node_set(&nodeset);
return 0;
}
```
在这个例子中,`main()`函数首先创建一个NUMA节点集,然后为每个节点创建一个新的线程,并使用`numa_bind()`将线程绑定到对应的节点。这有助于减少跨 NUMA 节点的数据迁移,提高程序性能。
阅读全文
相关推荐
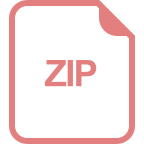
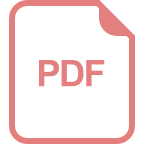
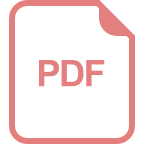
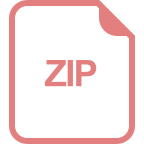
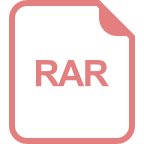
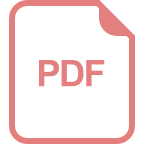
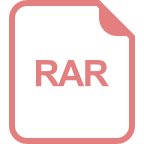
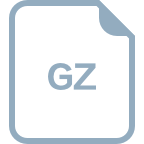
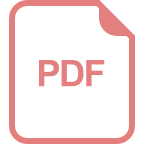
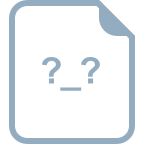
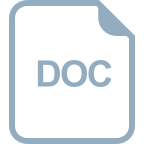
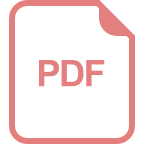
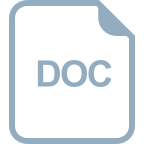
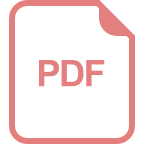
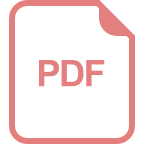