定义一个银行账户(BankAccount类),包括属性有帐号account和存款余额money,包含的方法有:构造、存款、取款、查询余额和显示帐号。创建若干账户类对象,完成存款等四个方法的测试。
时间: 2024-10-16 15:05:07 浏览: 162
好的,首先我们定义一个简单的`BankAccount`类,它包含了你提到的属性和方法:
```java
public class BankAccount {
private String account; // 账号
private double money; // 存款余额
// 构造函数初始化账号和余额
public BankAccount(String account, double initialMoney) {
this.account = account;
this.money = initialMoney;
}
// 存款方法,增加余额
public void deposit(double amount) {
if (amount > 0) {
money += amount;
}
}
// 取款方法,减少余额,如果余额不足则抛出异常
public void withdraw(double amount) throws InsufficientBalanceException {
if (amount <= money) {
money -= amount;
} else {
throw new InsufficientBalanceException("Insufficient balance.");
}
}
// 查询余额方法,返回当前的余额
public double checkBalance() {
return money;
}
// 显示账号方法,打印账号信息
@Override
public String toString() {
return "Account [" + account + ", Balance: " + money + "]";
}
}
```
接下来我们可以创建一些`BankAccount`对象并测试它们的功能:
```java
public static void main(String[] args) {
// 创建银行账户对象
BankAccount account1 = new BankAccount("123456", 1000);
BankAccount account2 = new BankAccount("789012", 2000);
// 测试存款方法
account1.deposit(500);
System.out.println(account1); // 输出 Account [123456, Balance: 1500]
// 测试取款方法
try {
account2.withdraw(2500);
} catch (InsufficientBalanceException e) {
System.out.println(e.getMessage()); // 输出 Insufficient balance.
}
System.out.println(account2); // 输出 Account [789012, Balance: 1750]
// 查询余额
System.out.printf("Account %s has a balance of %.2f%n", account1.getAccount(), account1.checkBalance());
System.out.printf("Account %s has a balance of %.2f%n", account2.getAccount(), account2.checkBalance());
}
```
阅读全文
相关推荐

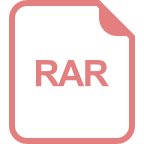














