用c++代码写出:以现实中银行卡为原型,进行需求调查,抽象出相应的类,设计类的成员函数和数据 成员,完成银行账户管理系统。编写程序,帮助工作人员有效、准确并且高校的完成存取事 件。(系统主要完成开户管理,存取钱管理,修改密码,注销等功能)
时间: 2024-06-09 12:04:24 浏览: 64
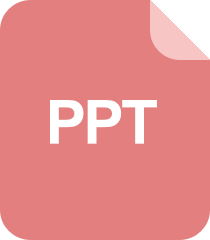
纯虚函数和抽象类-c++课件之继承-派生类
以下是一个简单的 C++ 代码示例,展示了如何根据银行卡的原型设计银行账户管理系统。
```
#include <iostream>
#include <string>
using namespace std;
class BankAccount {
public:
BankAccount(string name, string accountNumber, double balance) {
this->name = name;
this->accountNumber = accountNumber;
this->balance = balance;
}
void deposit(double amount) {
balance += amount;
}
void withdraw(double amount) {
if (balance >= amount) {
balance -= amount;
} else {
cout << "Insufficient funds!" << endl;
}
}
void changePassword(string newPassword) {
password = newPassword;
}
void closeAccount() {
closed = true;
}
void displayAccountInfo() {
cout << "Name: " << name << endl;
cout << "Account Number: " << accountNumber << endl;
cout << "Balance: " << balance << endl;
}
private:
string name;
string accountNumber;
double balance;
string password;
bool closed = false;
};
int main() {
BankAccount account("John Doe", "123456789", 1000.0);
account.displayAccountInfo();
// Deposit money
account.deposit(500.0);
account.displayAccountInfo();
// Withdraw money
account.withdraw(200.0);
account.displayAccountInfo();
// Change password
account.changePassword("newpassword");
account.displayAccountInfo();
// Close account
account.closeAccount();
account.displayAccountInfo();
return 0;
}
```
在这个代码示例中,我们首先定义了一个名为 `BankAccount` 的类,该类包含以下成员函数和数据成员:
- `BankAccount(string name, string accountNumber, double balance)`: 构造函数,用于创建一个新的银行账户对象,并传入名称、账户号码和初始余额参数。
- `void deposit(double amount)`: 存款函数,用于将一定金额的钱存入账户余额中。
- `void withdraw(double amount)`: 取款函数,用于从账户余额中取出一定金额的钱。
- `void changePassword(string newPassword)`: 修改密码函数,用于更改账户的密码。
- `void closeAccount()`: 注销账户函数,用于将账户标记为已注销。
- `void displayAccountInfo()`: 显示账户信息函数,用于打印账户的名称、账户号码和余额。
我们还定义了以下数据成员:
- `string name`: 账户名称。
- `string accountNumber`: 账户号码。
- `double balance`: 账户余额。
- `string password`: 账户密码。
- `bool closed`: 标记账户是否已注销。
在 `main` 函数中,我们首先创建了一个新的 `BankAccount` 对象,然后调用 `displayAccountInfo` 函数来显示账户信息。接下来,我们演示了如何使用 `deposit` 和 `withdraw` 函数进行存取款操作,使用 `changePassword` 函数修改密码,使用 `closeAccount` 函数注销账户,并使用 `displayAccountInfo` 函数显示账户信息。
阅读全文
相关推荐
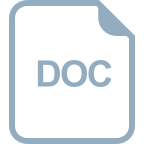
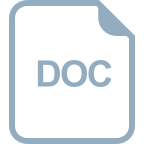


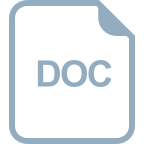
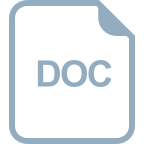
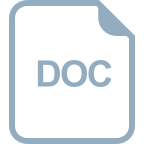
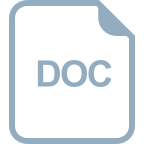
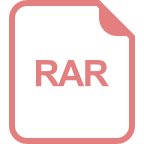
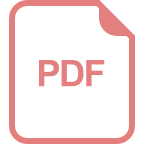
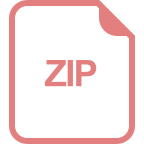
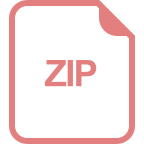
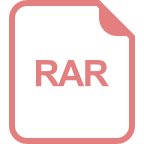
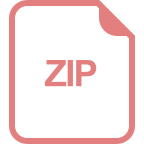