C++ 23种设计模式详细解释,还有代码演示
时间: 2023-11-14 14:23:25 浏览: 117
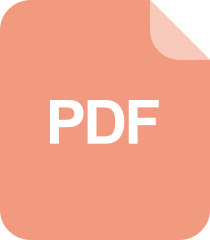
设计模式精解(GoF_23种设计解析附C__实现源码)
设计模式是一套被广泛接受的解决软件设计问题的方案。C++是一种广泛使用的编程语言,许多设计模式可以在C++中实现。下面是C++中23种设计模式的详细解释和代码演示。
1. 工厂方法模式(Factory Method Pattern)
工厂方法模式是一种创建型模式,它定义了一个用于创建对象的接口,但是由子类决定要实例化的类是哪一个。工厂方法让类把实例化推迟到子类。
代码演示:
```c++
class Product {
public:
virtual void use() = 0;
};
class ConcreteProduct : public Product {
public:
void use() override { std::cout << "Using ConcreteProduct" << std::endl; }
};
class Factory {
public:
virtual Product* createProduct() = 0;
};
class ConcreteFactory : public Factory {
public:
Product* createProduct() override { return new ConcreteProduct(); }
};
int main() {
Factory* factory = new ConcreteFactory();
Product* product = factory->createProduct();
product->use();
delete product;
delete factory;
return 0;
}
```
2. 抽象工厂模式(Abstract Factory Pattern)
抽象工厂模式是一种创建型模式,它提供了一个接口,用于创建相关或依赖对象的家族,而不需要明确指定具体类。
代码演示:
```c++
class Button {
public:
virtual void paint() = 0;
};
class WinButton : public Button {
public:
void paint() override { std::cout << "Painting a Windows button" << std::endl; }
};
class MacButton : public Button {
public:
void paint() override { std::cout << "Painting a Mac button" << std::endl; }
};
class CheckBox {
public:
virtual void paint() = 0;
};
class WinCheckBox : public CheckBox {
public:
void paint() override { std::cout << "Painting a Windows checkbox" << std::endl; }
};
class MacCheckBox : public CheckBox {
public:
void paint() override { std::cout << "Painting a Mac checkbox" << std::endl; }
};
class GUIFactory {
public:
virtual Button* createButton() = 0;
virtual CheckBox* createCheckBox() = 0;
};
class WinFactory : public GUIFactory {
public:
Button* createButton() override { return new WinButton(); }
CheckBox* createCheckBox() override { return new WinCheckBox(); }
};
class MacFactory : public GUIFactory {
public:
Button* createButton() override { return new MacButton(); }
CheckBox* createCheckBox() override { return new MacCheckBox(); }
};
int main() {
GUIFactory* factory;
#ifdef WIN_GUI
factory = new WinFactory();
#else
factory = new MacFactory();
#endif
Button* button = factory->createButton();
CheckBox* checkBox = factory->createCheckBox();
button->paint();
checkBox->paint();
delete checkBox;
delete button;
delete factory;
return 0;
}
```
3. 建造者模式(Builder Pattern)
建造者模式是一种创建型模式,它可以将一个复杂对象的构建与其表示分离,使得同样的构建过程可以创建不同的表示。
代码演示:
```c++
class Product {
public:
std::string part1_;
std::string part2_;
};
class Builder {
public:
virtual void buildPart1() = 0;
virtual void buildPart2() = 0;
virtual Product* getProduct() = 0;
};
class ConcreteBuilder : public Builder {
public:
void buildPart1() override { product_.part1_ = "Part 1"; }
void buildPart2() override { product_.part2_ = "Part 2"; }
Product* getProduct() override { return &product_; }
private:
Product product_;
};
class Director {
public:
void setBuilder(Builder* builder) { builder_ = builder; }
void construct() {
builder_->buildPart1();
builder_->buildPart2();
}
private:
Builder* builder_;
};
int main() {
ConcreteBuilder builder;
Director director;
director.setBuilder(&builder);
director.construct();
Product* product = builder.getProduct();
std::cout << "Product parts: " << product->part1_ << ", " << product->part2_ << std::endl;
return 0;
}
```
4. 原型模式(Prototype Pattern)
原型模式是一种创建型模式,它允许通过复制现有的对象来创建新的对象,而不是通过实例化来创建。这样做可以带来更好的性能和更少的代码。
代码演示:
```c++
class Prototype {
public:
virtual Prototype* clone() = 0;
virtual void use() = 0;
};
class ConcretePrototype : public Prototype {
public:
Prototype* clone() override { return new ConcretePrototype(*this); }
void use() override { std::cout << "Using ConcretePrototype" << std::endl; }
};
int main() {
Prototype* prototype = new ConcretePrototype();
Prototype* clone = prototype->clone();
prototype->use();
clone->use();
delete clone;
delete prototype;
return 0;
}
```
5. 单例模式(Singleton Pattern)
单例模式是一种创建型模式,它保证一个类只有一个实例,并提供一个全局访问点。
代码演示:
```c++
class Singleton {
public:
static Singleton* getInstance() {
if (instance_ == nullptr) {
instance_ = new Singleton();
}
return instance_;
}
void doSomething() { std::cout << "Doing something" << std::endl; }
private:
Singleton() {}
static Singleton* instance_;
};
Singleton* Singleton::instance_ = nullptr;
int main() {
Singleton* singleton = Singleton::getInstance();
singleton->doSomething();
return 0;
}
```
6. 适配器模式(Adapter Pattern)
适配器模式是一种结构型模式,它将一个类的接口转换成客户端所期望的另一种接口。适配器可以让原本不兼容的类一起工作。
代码演示:
```c++
class Target {
public:
virtual void request() = 0;
};
class Adaptee {
public:
void specificRequest() { std::cout << "Specific request" << std::endl; }
};
class Adapter : public Target {
public:
Adapter(Adaptee* adaptee) : adaptee_(adaptee) {}
void request() override { adaptee_->specificRequest(); }
private:
Adaptee* adaptee_;
};
int main() {
Adaptee* adaptee = new Adaptee();
Target* target = new Adapter(adaptee);
target->request();
delete target;
delete adaptee;
return 0;
}
```
7. 桥接模式(Bridge Pattern)
桥接模式是一种结构型模式,它将抽象部分与实现部分分离,使它们可以独立变化。桥接模式可以让一个类的实现细节对客户端透明。
代码演示:
```c++
class Implementor {
public:
virtual void operationImpl() = 0;
};
class ConcreteImplementorA : public Implementor {
public:
void operationImpl() override { std::cout << "ConcreteImplementorA" << std::endl; }
};
class ConcreteImplementorB : public Implementor {
public:
void operationImpl() override { std::cout << "ConcreteImplementorB" << std::endl; }
};
class Abstraction {
public:
Abstraction(Implementor* implementor) : implementor_(implementor) {}
virtual void operation() = 0;
protected:
Implementor* implementor_;
};
class RefinedAbstraction : public Abstraction {
public:
RefinedAbstraction(Implementor* implementor) : Abstraction(implementor) {}
void operation() override { implementor_->operationImpl(); }
};
int main() {
Implementor* implementorA = new ConcreteImplementorA();
Implementor* implementorB = new ConcreteImplementorB();
Abstraction* abstraction = new RefinedAbstraction(implementorA);
abstraction->operation();
delete abstraction;
abstraction = new RefinedAbstraction(implementorB);
abstraction->operation();
delete abstraction;
delete implementorA;
delete implementorB;
return 0;
}
```
8. 组合模式(Composite Pattern)
组合模式是一种结构型模式,它允许将对象组合成树形结构,以表示“部分-整体”的层次结构。组合模式可以让客户端统一处理单个对象和组合对象。
代码演示:
```c++
class Component {
public:
virtual ~Component() {}
virtual void operation() = 0;
};
class Leaf : public Component {
public:
void operation() override { std::cout << "Leaf operation" << std::endl; }
};
class Composite : public Component {
public:
void add(Component* component) { components_.push_back(component); }
void remove(Component* component) { components_.remove(component); }
void operation() override {
std::cout << "Composite operation" << std::endl;
for (auto component : components_) {
component->operation();
}
}
private:
std::list<Component*> components_;
};
int main() {
Component* leaf = new Leaf();
Component* composite = new Composite();
composite->add(leaf);
composite->operation();
delete composite;
delete leaf;
return 0;
}
```
9. 装饰器模式(Decorator Pattern)
装饰器模式是一种结构型模式,它允许在不改变对象的基础上动态地给对象添加功能。装饰器模式通过将对象放入包含其它对象的特殊容器中来实现这一功能。
代码演示:
```c++
class Component {
public:
virtual ~Component() {}
virtual void operation() = 0;
};
class ConcreteComponent : public Component {
public:
void operation() override { std::cout << "ConcreteComponent operation" << std::endl; }
};
class Decorator : public Component {
public:
Decorator(Component* component) : component_(component) {}
void operation() override { component_->operation(); }
private:
Component* component_;
};
class ConcreteDecoratorA : public Decorator {
public:
ConcreteDecoratorA(Component* component) : Decorator(component) {}
void operation() override {
Decorator::operation();
std::cout << "ConcreteDecoratorA operation" << std::endl;
}
};
class ConcreteDecoratorB : public Decorator {
public:
ConcreteDecoratorB(Component* component) : Decorator(component) {}
void operation() override {
Decorator::operation();
std::cout << "ConcreteDecoratorB operation" << std::endl;
}
};
int main() {
Component* component = new ConcreteComponent();
component = new ConcreteDecoratorA(component);
component = new ConcreteDecoratorB(component);
component->operation();
delete component;
return 0;
}
```
10. 外观模式(Facade Pattern)
外观模式是一种结构型模式,它提供了一个简单的接口,用于访问复杂系统中的一组接口。外观模式可以让客户端与子系统之间保持松散耦合。
代码演示:
```c++
class SubsystemA {
public:
void operationA() { std::cout << "Subsystem A operation" << std::endl; }
};
class SubsystemB {
public:
void operationB() { std::cout << "Subsystem B operation" << std::endl; }
};
class Facade {
public:
Facade() : subsystemA_(), subsystemB_() {}
void operation() {
subsystemA_.operationA();
subsystemB_.operationB();
}
private:
SubsystemA subsystemA_;
SubsystemB subsystemB_;
};
int main() {
Facade facade;
facade.operation();
return 0;
}
```
11. 享元模式(Flyweight Pattern)
享元模式是一种结构型模式,它通过共享尽可能多的数据来最小化内存使用。享元模式适用于需要大量相似对象的情况。
代码演示:
```c++
class Flyweight {
public:
virtual void operation() = 0;
};
class ConcreteFlyweight : public Flyweight {
public:
void operation() override { std::cout << "ConcreteFlyweight operation" << std::endl; }
};
class FlyweightFactory {
public:
static Flyweight* getFlyweight(int key) {
auto it = flyweights_.find(key);
if (it != flyweights_.end()) {
return it->second;
} else {
Flyweight* flyweight = new ConcreteFlyweight();
flyweights_[key] = flyweight;
return flyweight;
}
}
private:
static std::map<int, Flyweight*> flyweights_;
};
std::map<int, Flyweight*> FlyweightFactory::flyweights_;
int main() {
Flyweight* flyweight1 = FlyweightFactory::getFlyweight(1);
flyweight1->operation();
Flyweight* flyweight2 = FlyweightFactory::getFlyweight(1);
flyweight2->operation();
std::cout << std::boolalpha << (flyweight1 == flyweight2) << std::endl;
return 0;
}
```
12. 代理模式(Proxy Pattern)
代理模式是一种结构型模式,它提供了一个代理对象,以控制对另一个对象的访问。代理对象可以在访问者和实际对象之间充当中介。
代码演示:
```c++
class Subject {
public:
virtual void request() = 0;
};
class RealSubject : public Subject {
public:
void request() override { std::cout << "RealSubject request" << std::endl; }
};
class Proxy : public Subject {
public:
Proxy(Subject* subject) : subject_(subject) {}
void request() override { subject_->request(); }
private:
Subject* subject_;
};
int main() {
Subject* realSubject = new RealSubject();
Subject* proxy = new Proxy(realSubject);
proxy->request();
delete proxy;
delete realSubject;
return 0;
}
```
13. 责任链模式(Chain of Responsibility Pattern)
责任链模式是一种行为型模式,它允许多个对象都有机会处理请求,从而避免了请求者和接收者之间的耦合关系。责任链模式可以动态地组合处理对象。
代码演示:
```c++
class Handler {
public:
Handler(Handler* successor) : successor_(successor) {}
virtual void handleRequest(int request) {
if (successor_) {
successor_->handleRequest(request);
}
}
private:
Handler* successor_;
};
class ConcreteHandlerA : public Handler {
public:
ConcreteHandlerA(Handler* successor) : Handler(successor) {}
void handleRequest(int request) override {
if (request < 0) {
std::cout << "Handled by ConcreteHandlerA" << std::endl;
} else {
Handler::handleRequest(request);
}
}
};
class ConcreteHandlerB : public Handler {
public:
ConcreteHandlerB(Handler* successor) : Handler(successor) {}
void handleRequest(int request) override {
if (request > 0) {
std::cout << "Handled by ConcreteHandlerB" << std::endl;
} else {
Handler::handleRequest(request);
}
}
};
int main() {
Handler* handler = new ConcreteHandlerB(new ConcreteHandlerA(nullptr));
handler->handleRequest(-1);
handler->handleRequest(0);
handler->handleRequest(1);
delete handler;
return 0;
}
```
14. 命令模式(Command Pattern)
命令模式是一种行为型模式,它将请求封装为一个对象,从而允许您使用不同的请求、队列或日志来参数化其他对象。命令模式还支持可撤销的操作。
代码演示:
```c++
class Receiver {
public:
void action() { std::cout << "Receiver action" << std::endl; }
};
class Command {
public:
virtual ~Command() {}
virtual void execute() = 0;
};
class ConcreteCommand : public Command {
public:
ConcreteCommand(Receiver* receiver) : receiver_(receiver) {}
void execute() override { receiver_->action(); }
private:
Receiver* receiver_;
};
class Invoker {
public:
void setCommand(Command* command) { command_ = command; }
void executeCommand() { command_->execute(); }
private:
Command* command_;
};
int main() {
Receiver* receiver = new Receiver();
Command* command = new ConcreteCommand(receiver);
Invoker invoker;
invoker.setCommand(command);
invoker.executeCommand();
delete command;
delete receiver;
return 0;
}
```
15. 迭代器模式(Iterator Pattern)
迭代器模式是一种行为型模式,它提供了一种方法来访问集合对象的元素
阅读全文
相关推荐
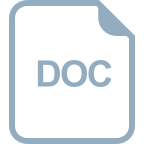
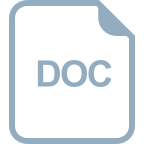
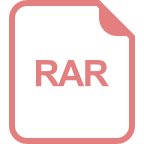
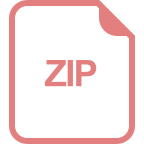
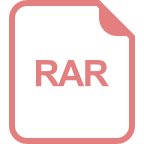
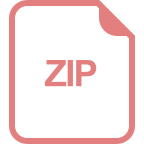
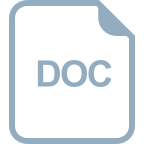
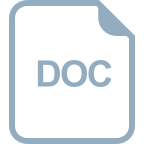
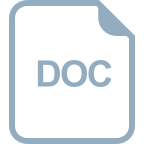
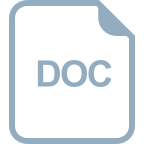
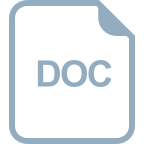
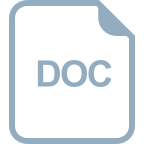
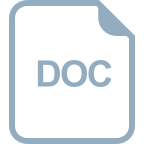
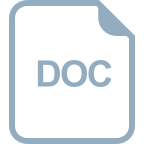
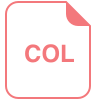

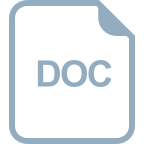
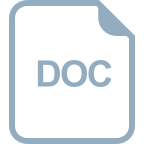