ffmpeg拆帧 C++
时间: 2024-10-15 19:03:17 浏览: 20
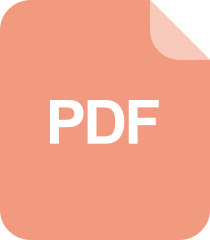
python ffmpeg任意提取视频帧的方法
FFmpeg是一个强大的跨平台多媒体处理工具库,特别适合于音频、视频文件的转换和编辑。在C++中使用FFmpeg进行视频拆帧,通常涉及到`libavcodec`库,这个库包含了视频编码和解码相关的功能。
首先,你需要包含必要的头文件,并链接FFmpeg库。例如:
```cpp
#include <opencv2/opencv.hpp> // 如果用于OpenCV集成
#include <fftw3.h>
#include <libavutil/imgutils.h> // 对于图像处理
#include <libavcodec/avcodec.h>
```
接下来,你可以创建AVCodecContext结构体来表示视频流,并打开视频文件:
```cpp
AVCodec * codec = avcodec_find_decoder(AV_CODEC_ID_H264); // 查找H.264解码器
AVCodecContext* ctx = avcodec_alloc_context3(codec);
if (!avcodec_open2(ctx, codec, NULL)) {
// 处理错误...
}
AVFrame frame;
av_frame_alloc(&frame, ctx->codec_id, ctx->width, ctx->height, ctx->pix_fmt);
```
然后读取视频帧并将其分离出来:
```cpp
int got_frame = 0;
while (av_read_frame(ctx, &packet) >= 0) {
if (pkt.stream_index == video_stream_idx) { // 检查是否为视频数据
AVPicture picture;
if (avpicture_fill(&picture, ctx->format, ctx->width, ctx->height, packet.data, packet.linesize[0])) {
// 处理图片数据...
av_frame_unref(frame);
got_frame = 0;
} else {
av_packet_unref(packet);
}
}
av_packet_unref(packet);
}
// 当所有帧都处理完后...
if (got_frame) {
av_frame_unref(frame);
}
avcodec_close(ctx);
```
最后别忘了释放资源:
```cpp
av_free(frame);
avcodec_free_context(&ctx);
```
阅读全文
相关推荐
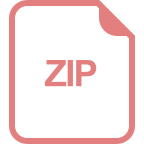
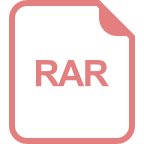















