C++驾校管理系统的车辆管理类代码
时间: 2024-09-19 15:10:07 浏览: 35
在创建一个简单的C++驾校管理系统中的车辆管理类(VehicleManager),我们通常会定义一个包含车的相关属性(如车牌号、型号、颜色等)以及方法(如添加车辆、删除车辆、查询车辆信息等)。以下是一个简化的示例:
```cpp
class Vehicle {
public:
// 车辆属性
std::string licensePlate;
std::string model;
std::string color;
// 构造函数
Vehicle(const std::string& plate, const std::string& m, const std::string& c)
: licensePlate(plate), model(m), color(c) {}
};
class VehicleManager {
private:
// 存储车辆的容器
std::vector<Vehicle> vehicles;
public:
// 添加车辆
void addVehicle(const std::string& plate, const std::string& model, const std::string& color) {
Vehicle newVehicle(plate, model, color);
vehicles.push_back(newVehicle);
}
// 删除车辆
void removeVehicle(const std::string& plate) {
for (auto it = vehicles.begin(); it != vehicles.end(); ++it) {
if (it->licensePlate == plate) {
vehicles.erase(it);
break;
}
}
}
// 查询车辆信息
std::optional<Vehicle> getVehicleByPlate(const std::string& plate) {
for (const auto& vehicle : vehicles) {
if (vehicle.licensePlate == plate) {
return vehicle;
}
}
return std::nullopt; // 如果未找到,返回空
}
};
// 使用示例
VehicleManager vm;
vm.addVehicle("AB1234", "Toyota Camry", "Blue");
if (const auto vehicle = vm.getVehicleByPlate("AB1234")) {
std::cout << "Found vehicle with plate " << vehicle.licensePlate << std::endl;
} else {
std::cout << "Vehicle not found." << std::endl;
}
```
阅读全文
相关推荐
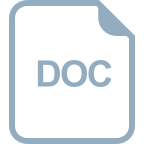
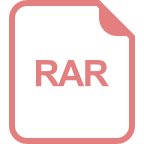
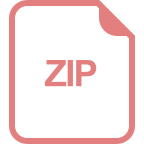







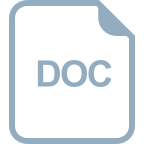
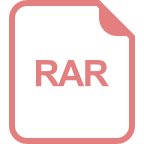
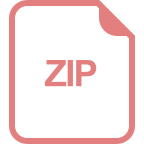
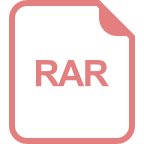
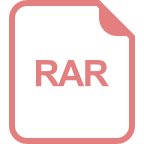
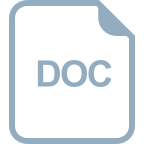
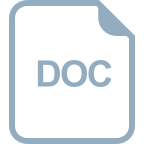
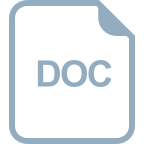
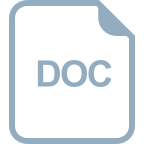