TypeError: list indices must be integers or slices, not getset_descriptor
时间: 2024-08-16 12:08:35 浏览: 85
这个错误提示 `TypeError: list indices must be integers or slices, not getset_descriptor` 意味着在Python中尝试访问列表元素时,使用的索引不是整数或切片(slice),而是获取/设置描述符(getset_descriptor)。描述符通常用于描述某些特殊类型的属性,比如类变量或元组中的元素,但在列表这类明确索引位置的数据结构中,索引应该是可以直接转换成整数的。
举个例子,如果你有这样一个情况:
```python
my_list = ['a', 'b', 'c']
my_list[my_list] # 这里my_list作为索引是不允许的
```
因为`my_list`不是一个可以作为索引的整数或切片,所以会抛出这个错误。修正这种情况需要确保索引是有效的整数:
```python
my_list = ['a', 'b', 'c']
correct_index = 0
print(my_list[correct_index]) # 正确的访问方式
```
相关问题
TypeError: list indices must be integers or slices, not list
这个错误通常是因为你试图使用一个列表来访问另一个列表中的元素,而你使用的是另一个列表作为索引,而不是整数或切片。请检查你的代码并确认你正在使用正确的索引类型。以下是一些可能导致此错误的代码示例:
```
my_list = [1, 2, 3]
index_list = [0, 1, 2]
# 错误的写法
my_list[index_list]
# 正确的写法
my_list[index_list[0]]
```
在上面的例子中,如果你尝试使用`index_list`来访问`my_list`中的元素,你会得到一个`TypeError`,因为`index_list`不是一个整数或切片。你需要使用`index_list`中的一个整数来访问`my_list`中的元素。
module TypeError: list indices must be integers or slices, not list
This error occurs when you try to use a list as an index for another list. In Python, only integers or slices (ranges) can be used as index values for lists. Here's an example of how this error can occur:
```
my_list = [1, 2, 3, 4]
my_index = [0, 1, 2]
# This line will raise a TypeError because my_index is a list
# and cannot be used as an index value for my_list
print(my_list[my_index])
```
To fix this error, make sure that you are using integer or slice values as index values for lists. For example:
```
my_list = [1, 2, 3, 4]
my_index = 2
# This line will print the value at index 2 of my_list (which is 3)
print(my_list[my_index])
```
阅读全文
相关推荐
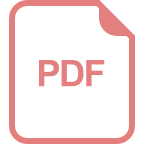
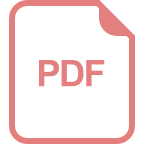








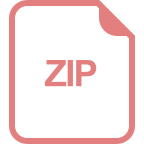
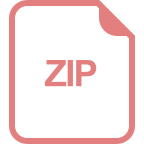