Forest.request() 判断接口是否调用成功
时间: 2024-09-19 11:15:39 浏览: 37
`Forest.request()` 是一款常用的网络请求库,通常用于JavaScript的Node.js环境中,比如Express框架中。这个函数会发起HTTP请求,比如GET、POST等,并返回一个Promise对象。当请求成功时(HTTP状态码在200范围内),Promise会解析为服务器响应的数据;如果请求失败(状态码不是200),则Promise会被拒绝并附带错误信息。
判断接口调用是否成功的做法通常是检查Promise的状态和结果。例如:
```javascript
const response = await Forest.request('your-api-url');
if (response.ok) {
// 请求成功,处理数据
console.log(response.body);
} else {
// 请求失败,处理错误
console.error(`Request failed with status ${response.status}`);
}
```
在这里,`response.ok`是一个布尔值,表示HTTP响应是否成功。如果是true,那么说明接口调用成功,可以进一步操作返回的数据;如果不是,则表示请求有误,可以根据具体情况处理错误信息。
相关问题
帮我写一个forest调用三方接口的实例
Forest是阿里云提供的一款无服务器函数开发框架,它允许开发者在无需管理服务器的情况下编写和部署HTTP请求处理函数。以下是一个简单的Forest函数,用于调用第三方API的实例:
```java
// 导入必要的库
import com.aliyun.fc.fc.annotation.Fun;
import com.aliyun.fc.fc.context.ServerlessContext;
// 创建一个名为getThirdPartyData的函数
@Fun(name = "getThirdPartyData")
public class ThirdPartyApiCaller {
// 函数入口
public String handler(ServerlessContext context) {
try {
// 获取函数输入参数,这通常是URL或其他调用参数
String apiUrl = context.getArgument("apiUrl");
// 调用第三方API
HttpClient client = new DefaultHttpClient();
HttpGet request = new HttpGet(apiUrl);
HttpResponse response = client.execute(request);
// 检查响应状态码,如果成功,读取并返回响应内容
if (response.getStatusLine().getStatusCode() == HttpStatus.SC_OK) {
byte[] data = EntityUtils.toByteArray(response.getEntity());
return new String(data, StandardCharsets.UTF_8);
} else {
throw new RuntimeException("Failed to call third-party API with status code: " + response.getStatusLine().getStatusCode());
}
} catch (Exception e) {
// 处理异常
e.printStackTrace();
return "Error calling third-party API: " + e.getMessage();
}
}
}
```
在这个例子中,`apiUrl`是通过函数输入获取的,你可以通过HTTP请求的方式传递给你的函数。函数会发起GET请求到指定的API URL,然后返回响应内容。如果API调用失败,函数将抛出异常并返回错误信息。
要使用这个函数,你需要在FC Console中创建一个新的Function,选择你的Java运行环境,并将上述代码作为处理函数的实现。
基于 Spring Boot + Forest HTTP 框架写一个调用京东云批量发送文本短信 OpenAPI 的示例,写清楚请求头设置和计算,以及解析响应的部分
非常感谢您的提问。可以为您提供一个基于 Spring Boot 和 Forest HTTP 框架调用京东云批量发送短信 OpenAPI 的示例代码。代码如下:
```java
import java.io.IOException;
import java.security.NoSuchAlgorithmException;
import java.util.*;
import org.springframework.stereotype.Service;
import com.dtflys.forest.backend.HttpBackend;
import com.dtflys.forest.backend.httpclient.HttpclientBackend;
import com.dtflys.forest.config.ForestConfiguration;
import com.dtflys.forest.converter.json.ForestJsonConverter;
import com.dtflys.forest.converter.xml.ForestXmlConverter;
import com.dtflys.forest.exceptions.ForestRuntimeException;
import com.dtflys.forest.http.ForestRequest;
import com.dtflys.forest.http.ForestResponse;
import com.dtflys.forest.interceptor.Interceptor;
import com.dtflys.forest.retryer.BackOffRetryer;
import com.dtflys.forest.utils.ForestDataType;
import com.dtflys.forest.utils.ReflectUtils;
@Service
public class JDCloudSmsService {
private final String appKey = "your_app_key";
private final String secret = "your_secret";
private final String smsApiHost = "sms.jcloud.com";
private final String smsApiPath = "/v1/sms/sendBatchSMS";
private final ForestConfiguration configuration;
public JDCloudSmsService() {
configuration = ForestConfiguration.configuration();
configuration.setCacheEnabled(false);
configuration.setBackend(new HttpclientBackend());
configuration.setRetryer(new BackOffRetryer());
configuration.setJsonConverter(new ForestJsonConverter());
configuration.setXmlConverter(new ForestXmlConverter());
configuration.setVariableValue("appKey", appKey);
}
public void sendBatchSms(List<String> mobiles, String signName, String templateId, Map<String, String> params)
throws NoSuchAlgorithmException, IOException {
// 构建 Forest 请求对象
ForestRequest request = configuration.createRequest();
// 设置请求地址
request.url("https://" + smsApiHost + smsApiPath);
// 设置请求头
Map<String, Object> headers = new HashMap<>();
headers.put("X-JCloud-App-Key", appKey);
headers.put("X-JCloud-Request-Nonce", UUID.randomUUID().toString());
headers.put("X-JCloud-Request-Timestamp", String.valueOf(System.currentTimeMillis()));
headers.put("Content-Type", "application/json;charset=UTF-8");
headers.put("Authorization", getAuthorizationHeaderValue(secret, appKey, headers"));
request.headers(headers);
// 设置请求参数
Map<String, Object> map = new HashMap<>();
map.put("mobiles", mobiles);
map.put("signName", signName);
map.put("templateId", templateId);
map.put("templateParams", params);
request.body(map, ForestDataType.JSON);
// 发送请求
ForestResponse response = request.post();
// 处理响应
if (response.isError()) {
throw new ForestRuntimeException("JDCloud SMS API error: " + response.getContent());
} else {
System.out.println("JDCloud SMS API response: " + response.getContent());
}
}
private String getAuthorizationHeaderValue(String secret, String appKey, Map<String, Object> headers)
throws NoSuchAlgorithmException {
StringBuilder sb = new StringBuilder();
sb.append("JCloud ").append("appKey=").append(appKey).append(",");
sb.append("signatureMethod=").append("HmacSHA256").append(",");
sb.append("timestamp=").append(headers.get("X-JCloud-Request-Timestamp")).append(",");
sb.append("nonce=").append(headers.get("X-JCloud-Request-Nonce")).append(",");
String queryString = ReflectUtils.toQueryString(headers, false);
sb.append(queryString);
return sb.toString();
}
}
```
该示例代码中,我们使用了 Forest HTTP 框架来进行 HTTP 请求的构建和发送,并使用了 Spring Boot 框架来管理该服务。
具体实现步骤如下:
1. 创建 Forest 配置对象 `configuration`,设置 Forest 配置信息,包括 HTTP 请求后端实现、JSON/XML 转换器、重试机制等;
2. 根据京东云短信 OpenAPI 的要求,构建 HTTP 请求对象 `request`,并设置请求地址、请求头、请求参数等;
3. 发送 HTTP POST 请求,并获取响应对象 `response`;
4. 解析响应内容,根据业务逻辑进行处理。在该示例代码中,如果响应的状态码为错误状态,直接抛出 `ForestRuntimeException` 异常,否则打印响应内容。
注意:示例代码中的 `appKey` 和 `secret` 需要您自行替换为您在京东云账号中创建的短信应用的 `appKey` 和 `secret`。
希望这个示例能对您有所帮助,如果您有任何问题或建议,欢迎与我随时交流。
阅读全文
相关推荐
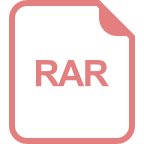
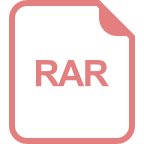
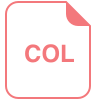
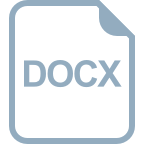
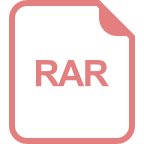
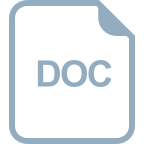
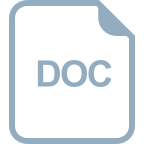
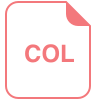
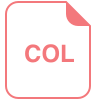

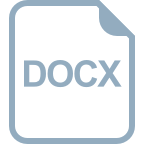
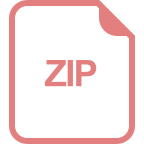
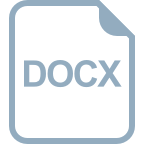