C代码手动设置两个年月日时间,计算这两个时间相差的天数,不用time.h函数
时间: 2024-10-14 17:03:49 浏览: 30
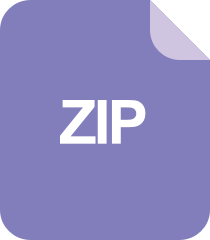
计算两个时间相差年月日时分秒.zip

在C语言中,如果你想要手动设置两个年月日的时间并计算它们之间的天数,你可以创建结构体来存储日期,并编写自定义的加减法函数来处理日期。以下是一个简单的示例:
```c
#include <stdio.h>
// 定义日期结构体
typedef struct {
int year;
int month;
int day;
} Date;
// 计算两个日期之间相差的天数
int date_difference(Date d1, Date d2) {
// 首先判断第一个日期是否小于第二个日期
if (d1.year > d2.year || (d1.year == d2.year && (d1.month > d2.month || (d1.month == d2.month && d1.day >= d2.day))))
return -1; // 如果第一个日期晚于第二个,则直接返回负数
// 分别计算年份、月份和天数的差值
int years = d2.year - d1.year;
int months_in_first_year = d1.month + years * 12;
int days_in_years = years * 365; // 简单计算每年天数(假设无闰年)
int months_days = (months_in_first_year - 1) * 30; // 估算每个月的平均天数
// 加上剩余月份和当前月份的天数差
int remaining_days = (d2.month - d1.month) * 30 + d2.day - d1.day;
// 考虑闰年的影响(每四年一次,百年不闰,四百整百年闰)
if (years >= 4 && (years % 100 != 0 || years % 400 == 0)) {
days_in_years += 1; // 闰年增加一天
}
// 返回总天数差
return days_in_years + months_days + remaining_days;
}
int main() {
Date date1 = {2022, 10, 1};
Date date2 = {2023, 5, 15};
int difference = date_difference(date1, date2);
printf("The difference between the two dates is %d days.\n", difference);
return 0;
}
```
这个示例代码仅作演示,它并未考虑所有边缘情况和精确的闰年规则。在实际应用中,你可能需要引入更复杂的算法来处理闰年问题。
阅读全文
相关推荐
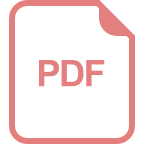
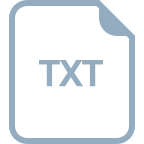


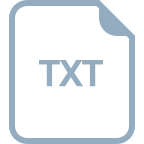


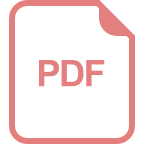
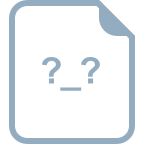
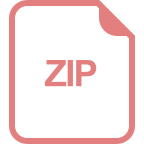
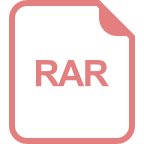
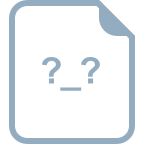
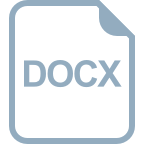
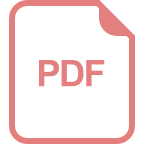
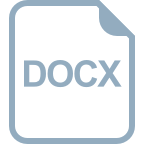