背包问题用类封装快速排序代码C++
时间: 2024-11-03 08:13:58 浏览: 32
背包问题通常涉及选择物品来最大化背包能够容纳的价值,而不超过其容量限制。在C++中,可以使用动态规划结合类封装的方式来解决这个问题。下面是一个简单的模拟背包问题的类封装和快速排序示例,这个例子假设我们有一个包含物品(每个物品有自己的价值和重量)的数据结构,并且我们需要找到一种组合,使得物品总重量不超过背包容量的同时,总价值最大。
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
class Item {
public:
int value;
int weight;
// 构造函数
Item(int v, int w) : value(v), weight(w) {}
};
class KnapsackProblem {
private:
std::vector<Item> items;
int capacity;
public:
KnapsackProblem(std::vector<Item>& items, int c) : items(items), capacity(c) {}
int max_value(int index) {
if (index == items.size() || capacity <= 0)
return 0;
// 快速排序辅助方法,这里简化处理
std::sort(items.begin() + index, items.end(), [](const Item& a, const Item& b) { return a.weight < b.weight; });
// 分治法策略
int best_with = max_value(index + 1); // 不选当前物品
int best_with_item = max_value(index + 1) + items[index].value; // 选当前物品
return std::max(best_with, best_with_item);
}
int knapsack() {
return max_value(0);
}
};
int main() {
std::vector<Item> items = { {60, 10}, {100, 20}, {120, 30} }; // 示例物品列表
int capacity = 50; // 背包容量
KnapsackProblem kp(items, capacity);
int maxValue = kp.knapsack();
std::cout << "Max value with " << capacity << " units: " << maxValue << std::endl;
return 0;
}
阅读全文
相关推荐



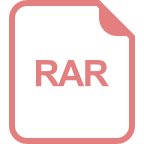

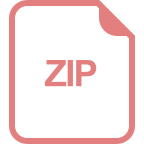



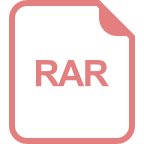








