python画烟花绽放出字
时间: 2023-11-16 19:00:13 浏览: 158
这篇文章介绍了如何使用Python制作一个炫酷的烟花秀,并在烟花绽放时显示文字。在代码中,使用了pygame库来创建游戏窗口和绘制图形,同时使用了字体文件来渲染文字。具体实现方法如下:
1.在代码中导入pygame库和random库。
2.定义一个Firework类,用于创建烟花对象。在该类中,定义了烟花的颜色、位置、速度等属性,并实现了烟花绽放的动画效果。
3.定义一个update函数,用于更新烟花的状态并绘制到屏幕上。在该函数中,遍历所有的烟花对象,更新它们的状态并绘制到屏幕上。
4.在主函数中,初始化pygame环境,设置背景图片、文字等,并创建两个烟花对象。然后进入主循环,每隔60ms刷新一次屏幕,绘制背景、文字和烟花,并根据一定的概率创建新的烟花对象。
5.在Firework类中添加一个方法,用于在烟花绽放时显示文字。具体实现方法是,在烟花绽放的过程中,根据烟花的位置和颜色创建一个Surface对象,并使用字体文件渲染文字。然后将该Surface对象绘制到屏幕上。
相关问题
Python模拟烟花绽放
好的,下面是一个简单的Python程序,可以模拟烟花绽放的效果:
```python
import random
import time
import turtle
# 设置画布大小
turtle.screensize(800, 600)
# 设置画笔大小
turtle.pensize(3)
# 定义烟花绽放函数
def firework(x, y):
turtle.up()
turtle.goto(x, y)
turtle.down()
# 画出烟花的线
turtle.color("#FFFF33")
turtle.fd(50)
# 画出烟花的花心
turtle.color("#FF66FF")
turtle.begin_fill()
turtle.circle(10)
turtle.end_fill()
# 画出烟花的花瓣
colors = ["#FF3333", "#FF9933", "#FFFF33", "#66FF33", "#33FFCC", "#6666FF", "#FF33FF"]
for i in range(36):
turtle.color(random.choice(colors))
turtle.up()
turtle.goto(x, y)
turtle.down()
turtle.fd(50)
turtle.right(10)
# 隐藏画笔
turtle.hideturtle()
# 循环绘制烟花
while True:
x = random.randint(-400, 400)
y = random.randint(-200, 200)
firework(x, y)
time.sleep(1)
turtle.done()
```
以上代码使用了Python的turtle库,通过随机生成烟花的位置和颜色来模拟烟花绽放的效果。你可以尝试运行这个程序来观察效果。
python烟花绽放
以下是Python模拟烟花绽放的代码示例:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置屏幕大小和标题
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Fireworks")
# 定义烟花类
class Firework:
def __init__(self):
self.x = random.randint(0, 800) # 烟花的x坐标
self.y = 600 # 烟花的y坐标
self.color = (random.randint(0, 255), random.randint(0,255), random.randint(0, 255)) # 烟花的颜色
self.speed = random.randint(5, 20) # 烟花的速度
self.exploded = False # 烟花是否已经爆炸
self.particles = [] # 烟花爆炸后的粒子
def launch(self):
if not self.exploded:
self.y -= self.speed # 烟花向上移动
if self.y <= random.randint(100, 200): # 烟花到达顶部后爆炸
self.explode()
def explode(self):
self.exploded = True
for i in range(100): # 生成100个粒子
self.particles.append(Particle(self.x, self.y, self.color))
def show(self):
if not self.exploded:
pygame.draw.circle(screen, self.color, (self.x, int(self.y)), 5)
else:
for particle in self.particles:
particle.show()
# 定义粒子类
class Particle:
def __init__(self, x, y, color):
self.x = x # 粒子的x坐标
self.y = y # 粒子的y坐标
self.color = color # 粒子的颜色
self.speed = random.randint(1, 5) # 粒子的速度
self.direction = random.randint(0, 360) # 粒子的方向
self.radius = random.randint(2, 5) # 粒子的半径
self.alpha = 255 # 粒子的透明度
def move(self):
self.x += self.speed * math.cos(math.radians(self.direction)) # 粒子的x方向移动
self.y -= self.speed * math.sin(math.radians(self.direction)) # 粒子的y方向移动
self.alpha -= 5 # 粒子的透明度减少
def show(self):
pygame.draw.circle(screen, (self.color[0], self.color[1], self.color[2], self.alpha), (int(self.x), int(self.y)), self.radius)
def finished(self):
return self.alpha <= 0
# 创建烟花列表
fireworks = []
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
# 按下 1 时生成一个新的烟花
if event.key == pygame.K_1:
fireworks.append(Firework())
# 按下 2 时生成 10 个烟花
if event.key == pygame.K_2:
for i in range(10):
fireworks.append(Firework())
# 填充背景色
screen.fill((0, 0, 0))
# 更新烟花状态
for firework in fireworks:
firework.launch()
firework.show()
# 更新粒子状态
for firework in fireworks:
if firework.exploded:
for particle in firework.particles:
particle.move()
particle.show()
if particle.finished():
firework.particles.remove(particle)
# 更新屏幕
pygame.display.update()
# 退出pygame
pygame.quit()
```
相关推荐
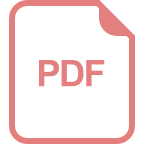












