python烟花绽放
时间: 2023-11-24 07:53:25 浏览: 143
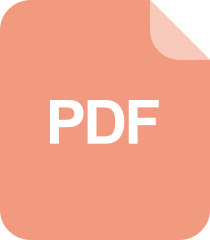
新年快乐! python实现绚烂的烟花绽放效果

以下是Python模拟烟花绽放的代码示例:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置屏幕大小和标题
screen = pygame.display.set_mode((800, 600))
pygame.display.set_caption("Fireworks")
# 定义烟花类
class Firework:
def __init__(self):
self.x = random.randint(0, 800) # 烟花的x坐标
self.y = 600 # 烟花的y坐标
self.color = (random.randint(0, 255), random.randint(0,255), random.randint(0, 255)) # 烟花的颜色
self.speed = random.randint(5, 20) # 烟花的速度
self.exploded = False # 烟花是否已经爆炸
self.particles = [] # 烟花爆炸后的粒子
def launch(self):
if not self.exploded:
self.y -= self.speed # 烟花向上移动
if self.y <= random.randint(100, 200): # 烟花到达顶部后爆炸
self.explode()
def explode(self):
self.exploded = True
for i in range(100): # 生成100个粒子
self.particles.append(Particle(self.x, self.y, self.color))
def show(self):
if not self.exploded:
pygame.draw.circle(screen, self.color, (self.x, int(self.y)), 5)
else:
for particle in self.particles:
particle.show()
# 定义粒子类
class Particle:
def __init__(self, x, y, color):
self.x = x # 粒子的x坐标
self.y = y # 粒子的y坐标
self.color = color # 粒子的颜色
self.speed = random.randint(1, 5) # 粒子的速度
self.direction = random.randint(0, 360) # 粒子的方向
self.radius = random.randint(2, 5) # 粒子的半径
self.alpha = 255 # 粒子的透明度
def move(self):
self.x += self.speed * math.cos(math.radians(self.direction)) # 粒子的x方向移动
self.y -= self.speed * math.sin(math.radians(self.direction)) # 粒子的y方向移动
self.alpha -= 5 # 粒子的透明度减少
def show(self):
pygame.draw.circle(screen, (self.color[0], self.color[1], self.color[2], self.alpha), (int(self.x), int(self.y)), self.radius)
def finished(self):
return self.alpha <= 0
# 创建烟花列表
fireworks = []
# 游戏循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
# 按下 1 时生成一个新的烟花
if event.key == pygame.K_1:
fireworks.append(Firework())
# 按下 2 时生成 10 个烟花
if event.key == pygame.K_2:
for i in range(10):
fireworks.append(Firework())
# 填充背景色
screen.fill((0, 0, 0))
# 更新烟花状态
for firework in fireworks:
firework.launch()
firework.show()
# 更新粒子状态
for firework in fireworks:
if firework.exploded:
for particle in firework.particles:
particle.move()
particle.show()
if particle.finished():
firework.particles.remove(particle)
# 更新屏幕
pygame.display.update()
# 退出pygame
pygame.quit()
```
阅读全文
相关推荐
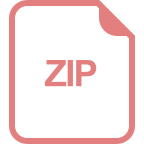















