C++实现链表插入删除查找
时间: 2024-09-29 08:10:29 浏览: 5
在C++中,链表是一种常见的数据结构,它由一系列节点组成,每个节点包含数据和指向下一个节点的指针。以下是基本的链表操作:
1. 插入元素:
- **在头部插入** (`insertAtBeginning`): 创建新节点并将其指针设置为头节点,然后将当前头节点的指针更新为新节点。
```cpp
struct Node {
int data;
Node* next;
};
void insertAtBeginning(Node*& head, int value) {
Node* newNode = new Node{value, nullptr};
newNode->next = head;
head = newNode;
}
```
- **在指定位置插入** (`insertAtPosition`): 需遍历找到目标位置,并调整后续节点的指针。
```cpp
void insertAtPosition(Node*& head, int position, int value) {
if (position <= 0) return; // 非法位置处理
Node* newNode = new Node{value, nullptr};
Node* current = head;
for (int i = 0; i < position - 1 && current != nullptr; ++i) {
current = current->next;
}
newNode->next = current->next;
if (current != nullptr) current->next = newNode;
}
```
2. 删除元素:
- **删除头节点** (`removeHead`): 如果头节点存在,则更新头节点为第二个节点。
```cpp
void removeHead(Node*& head) {
if (head != nullptr) {
Node* temp = head;
head = head->next;
delete temp;
} else {
std::cout << "List is empty" << std::endl;
}
}
```
- **根据值删除** (`removeValue`): 遍历链表寻找特定值的节点并删除。
```cpp
void removeValue(Node*& head, int value) {
while (head != nullptr && head->data == value) {
Node* temp = head;
head = head->next;
delete temp;
}
Node* current = head;
while (current != nullptr && current->next != nullptr) {
if (current->next->data == value) {
Node* toDelete = current->next;
current->next = current->next->next;
delete toDelete;
} else {
current = current->next;
}
}
}
```
3. 查找元素:
- **线性查找** (`search`): 遍历整个链表直到找到匹配项或结束。
```cpp
bool search(Node* head, int value) {
Node* current = head;
while (current != nullptr) {
if (current->data == value) return true;
current = current->next;
}
return false;
}
```
相关推荐
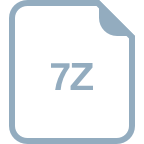
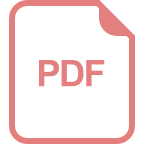
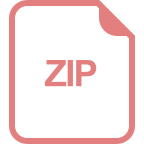














